Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Connect to Databricks Data from Blazor Apps
Build ASP.NET Core Blazor C# apps that integrate with real-time Databricks data using standard SQL.
Blazor is a framework for developing modern, client-side web UIs using .NET technology. Instead of coding in JavaScript, developers can use the familiar C# language and .NET libraries to build app UIs.
The CData ADO.NET Provider for Databricks can be used with standard ADO.NET interfaces, such as LINQ and Entity Framework, to interact with live Databricks data. Since Blazor supports .NET Core, developers can use CData ADO.NET Providers in Blazor apps. In this article, we will guide you to build a simple Blazor app that talks to Databricks using standard SQL queries.
About Databricks Data Integration
Accessing and integrating live data from Databricks has never been easier with CData. Customers rely on CData connectivity to:
- Access all versions of Databricks from Runtime Versions 9.1 - 13.X to both the Pro and Classic Databricks SQL versions.
- Leave Databricks in their preferred environment thanks to compatibility with any hosting solution.
- Secure authenticate in a variety of ways, including personal access token, Azure Service Principal, and Azure AD.
- Upload data to Databricks using Databricks File System, Azure Blog Storage, and AWS S3 Storage.
While many customers are using CData's solutions to migrate data from different systems into their Databricks data lakehouse, several customers use our live connectivity solutions to federate connectivity between their databases and Databricks. These customers are using SQL Server Linked Servers or Polybase to get live access to Databricks from within their existing RDBMs.
Read more about common Databricks use-cases and how CData's solutions help solve data problems in our blog: What is Databricks Used For? 6 Use Cases.
Getting Started
Install the CData ADO.NET Provider for Databricks
CData ADO.NET Providers allow users to access Databricks just like they would access SQL Server, using simple SQL queries.
Install the Databricks ADO.NET Data Provider from the CData website or from NuGet. Search NuGet for "Databricks ADO.NET Data Provider."
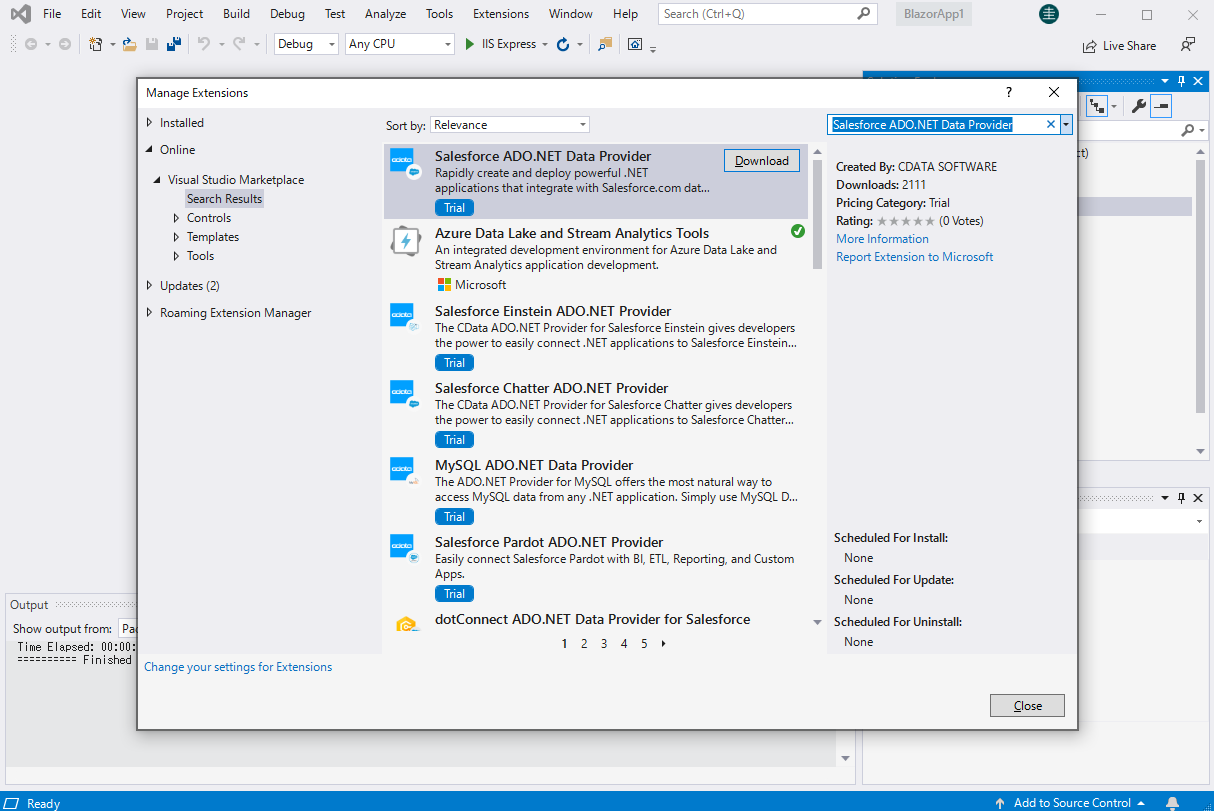
Create a Databricks-Connected Blazor App
Start by creating a Blazor project that references the CData ADO.NET Provider for Databricks
- Create a Blazor project on Visual Studio.
- From the Solution Explorer, right click Dependencies, then click Add Project Reference.
- In the Reference Manager, click the Browse button, and choose the .dll file of the installed ADO.NET Provider (e.g. System.Data.CData.Databricks.dll, typically located at C:\Program Files\CData\CData ADO.NET Provider for Databricks\lib etstandard2.0).
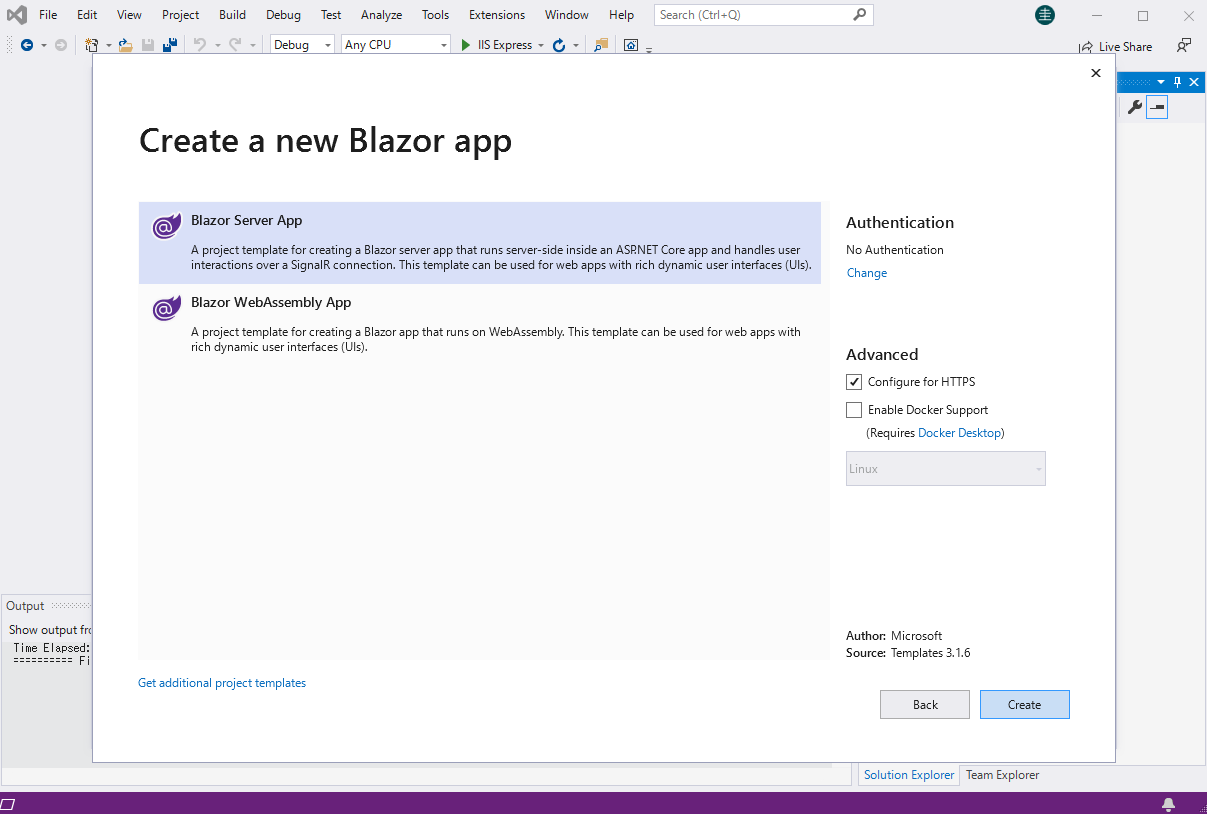
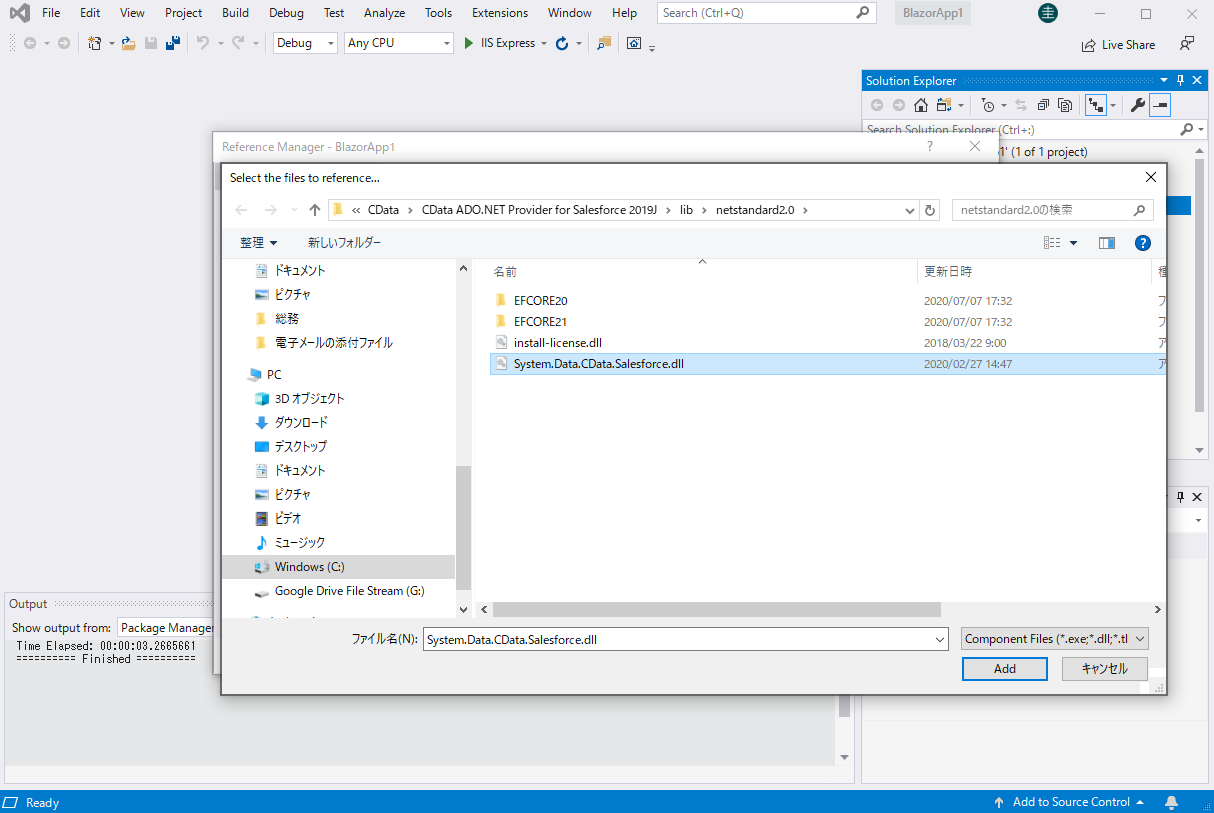
SELECT Databricks Data from the Blazor App
- Open the Index.razor file from the Project page.
- In a DatabricksConnection object, set the connection string:
To connect to a Databricks cluster, set the properties as described below.
Note: The needed values can be found in your Databricks instance by navigating to Clusters, and selecting the desired cluster, and selecting the JDBC/ODBC tab under Advanced Options.
- Server: Set to the Server Hostname of your Databricks cluster.
- HTTPPath: Set to the HTTP Path of your Databricks cluster.
- Token: Set to your personal access token (this value can be obtained by navigating to the User Settings page of your Databricks instance and selecting the Access Tokens tab).
For example: Server=127.0.0.1;Port=443;TransportMode=HTTP;HTTPPath=MyHTTPPath;UseSSL=True;User=MyUser;Password=MyPassword;
- The code below creates a simple Blazor app for displaying Databricks data, using standard SQL to query Databricks just like SQL Server.
@page "/" @using System.Data; @using System.Data.CData.Databricks; <h1>Hello, world!</h1> Welcome to your Data app. <div class="row"> <div class="col-12"> @using (DatabricksConnection connection = new DatabricksConnection( "Server=127.0.0.1;Port=443;TransportMode=HTTP;HTTPPath=MyHTTPPath;UseSSL=True;User=MyUser;Password=MyPassword;")) { var sql = "SELECT City, CompanyName FROM Customers WHERE Country = 'US'"; var results = new DataTable(); DatabricksDataAdapter dataAdapter = new DatabricksDataAdapter(sql, connection); dataAdapter.Fill(results); <table class="table table-bordered"> <thead class="thead-light"> <tr> @foreach (DataColumn item in results.Rows[0].Table.Columns) { <th scope="col">@item.ColumnName</th> } </tr> </thead> <tbody> @foreach (DataRow row in results.Rows) { <tr> @foreach (var column in row.ItemArray) { <td>@column.ToString()</td> } </tr> } </tbody> </table> } </div> </div>
- Rebuild and run the project. The ADO.NET Provider renders Databricks data as an HTML table in the Blazor app.
At this point, you have a Databricks-connected Blazor app, capable of working with live Databricks data just like you would work with a SQL Server instance. Download a free, 30-day trial and start working with live Databricks data in your Blazor apps today.