Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Connect to Act-On Data from Blazor Apps
Build ASP.NET Core Blazor C# apps that integrate with real-time Act-On data using standard SQL.
Blazor is a framework for developing modern, client-side web UIs using .NET technology. Instead of coding in JavaScript, developers can use the familiar C# language and .NET libraries to build app UIs.
The CData ADO.NET Provider for Act-On can be used with standard ADO.NET interfaces, such as LINQ and Entity Framework, to interact with live Act-On data. Since Blazor supports .NET Core, developers can use CData ADO.NET Providers in Blazor apps. In this article, we will guide you to build a simple Blazor app that talks to Act-On using standard SQL queries.
Install the CData ADO.NET Provider for Act-On
CData ADO.NET Providers allow users to access Act-On just like they would access SQL Server, using simple SQL queries.
Install the Act-On ADO.NET Data Provider from the CData website or from NuGet. Search NuGet for "Act-On ADO.NET Data Provider."
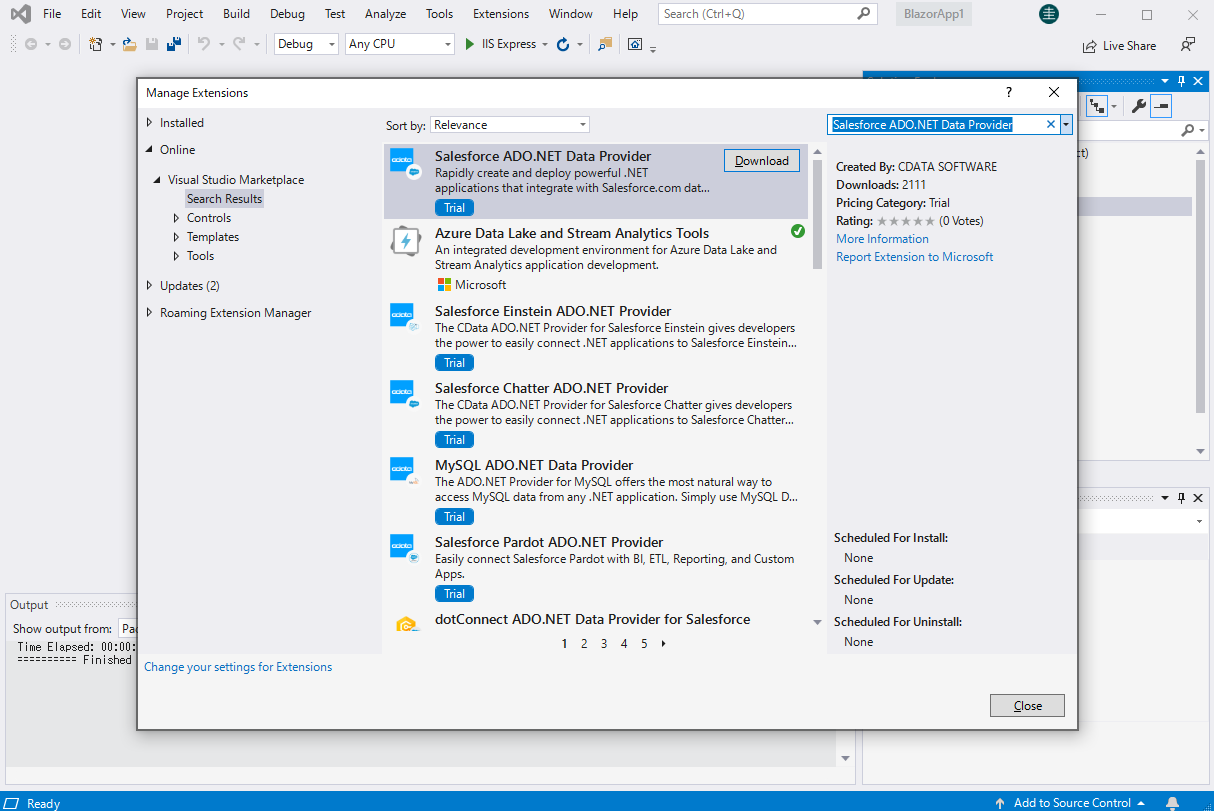
Create a Act-On-Connected Blazor App
Start by creating a Blazor project that references the CData ADO.NET Provider for Act-On
- Create a Blazor project on Visual Studio.
- From the Solution Explorer, right click Dependencies, then click Add Project Reference.
- In the Reference Manager, click the Browse button, and choose the .dll file of the installed ADO.NET Provider (e.g. System.Data.CData.ActOn.dll, typically located at C:\Program Files\CData\CData ADO.NET Provider for Act-On\lib etstandard2.0).
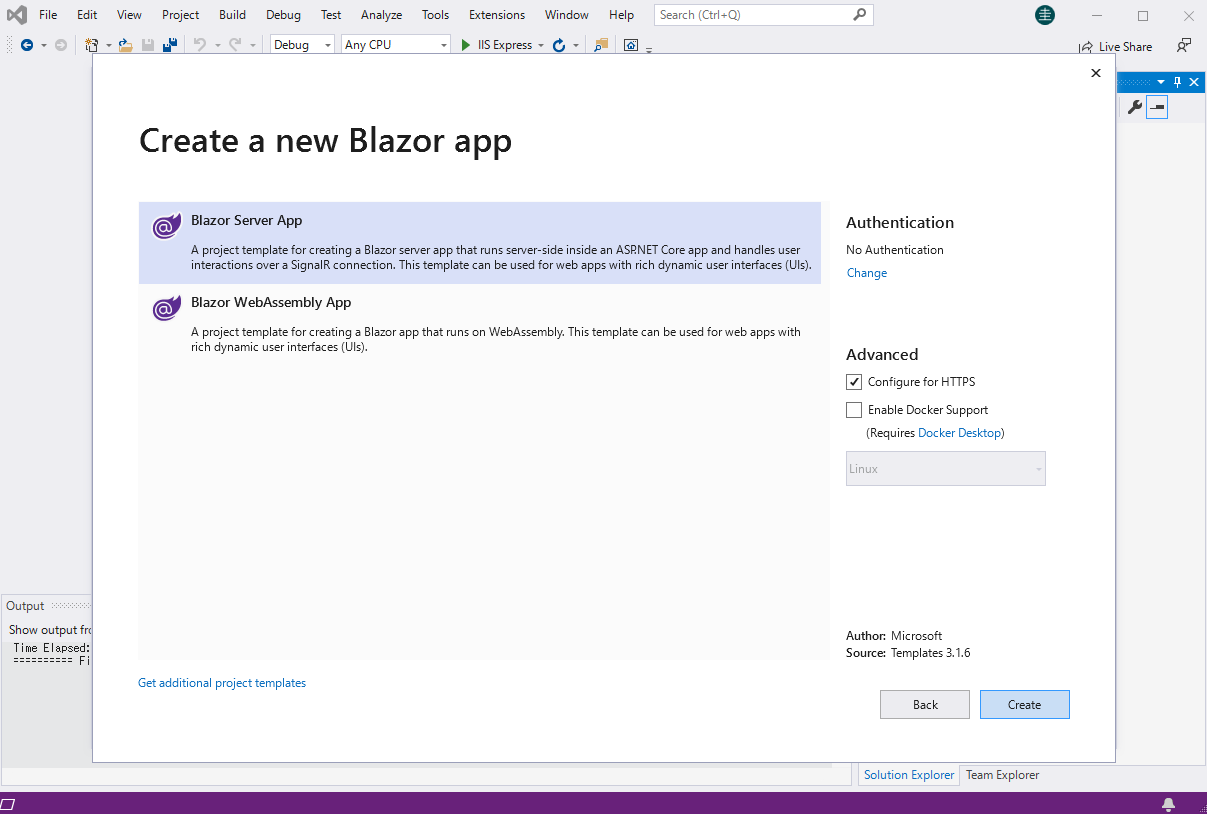
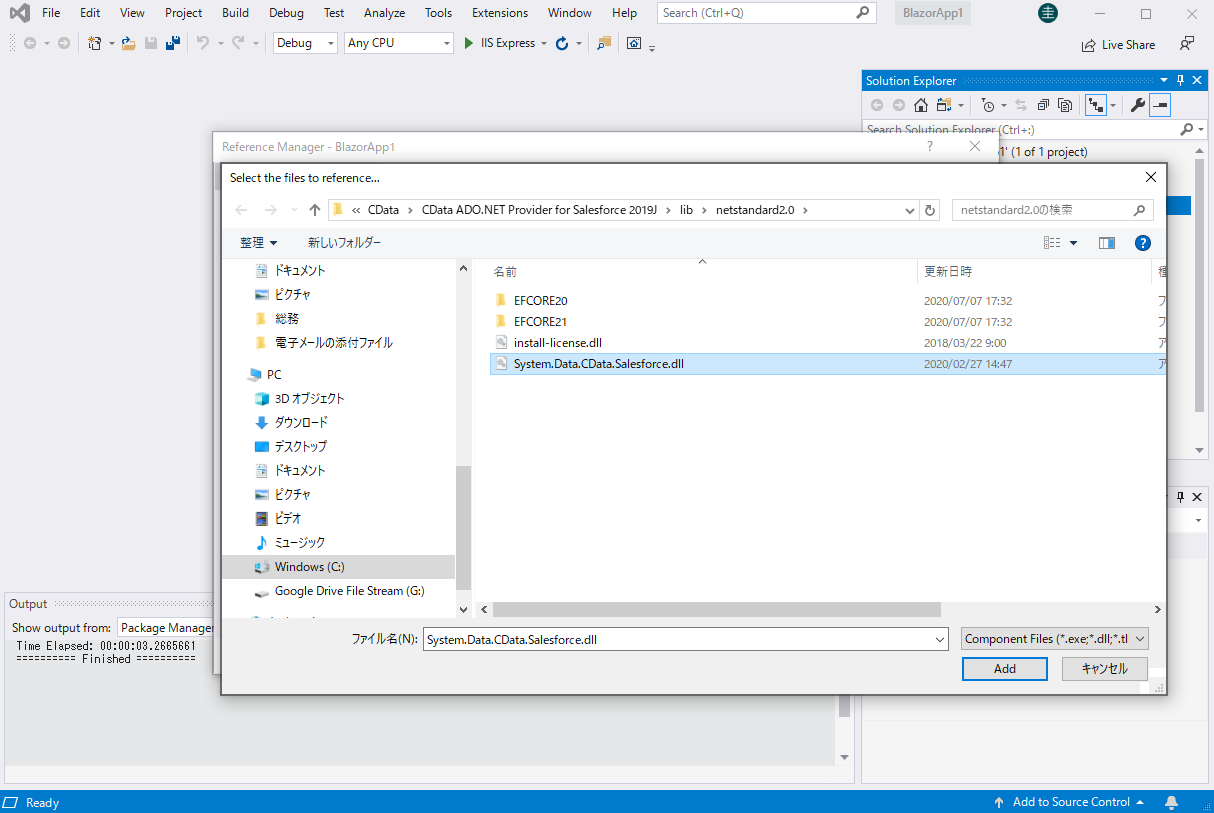
SELECT Act-On Data from the Blazor App
- Open the Index.razor file from the Project page.
- In a ActOnConnection object, set the connection string:
ActOn uses the OAuth authentication standard. To authenticate using OAuth, you will need to create an app to obtain the OAuthClientId, OAuthClientSecret, and CallbackURL connection properties.
See the Getting Started guide in the CData driver documentation for more information.
For example:
- The code below creates a simple Blazor app for displaying Act-On data, using standard SQL to query Act-On just like SQL Server.
@page "/" @using System.Data; @using System.Data.CData.ActOn; <h1>Hello, world!</h1> Welcome to your Data app. <div class="row"> <div class="col-12"> @using (ActOnConnection connection = new ActOnConnection( "")) { var sql = "SELECT Id, Name FROM Images"; var results = new DataTable(); ActOnDataAdapter dataAdapter = new ActOnDataAdapter(sql, connection); dataAdapter.Fill(results); <table class="table table-bordered"> <thead class="thead-light"> <tr> @foreach (DataColumn item in results.Rows[0].Table.Columns) { <th scope="col">@item.ColumnName</th> } </tr> </thead> <tbody> @foreach (DataRow row in results.Rows) { <tr> @foreach (var column in row.ItemArray) { <td>@column.ToString()</td> } </tr> } </tbody> </table> } </div> </div>
- Rebuild and run the project. The ADO.NET Provider renders Act-On data as an HTML table in the Blazor app.
At this point, you have a Act-On-connected Blazor app, capable of working with live Act-On data just like you would work with a SQL Server instance. Download a free, 30-day trial and start working with live Act-On data in your Blazor apps today.