Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Use Dash to Build to Web Apps on Typeform Data
Create Python applications that use pandas and Dash to build Typeform-connected web apps.
The rich ecosystem of Python modules lets you get to work quickly and integrate your systems more effectively. With the CData API Driver for Python, the pandas module, and the Dash framework, you can build Typeform-connected web applications for Typeform data. This article shows how to connect to Typeform with the CData Connector and use pandas and Dash to build a simple web app for visualizing Typeform data.
With built-in, optimized data processing, the CData Python Connector offers unmatched performance for interacting with live Typeform data in Python. When you issue complex SQL queries from Typeform, the driver pushes supported SQL operations, like filters and aggregations, directly to Typeform and utilizes the embedded SQL engine to process unsupported operations client-side (often SQL functions and JOIN operations).
Connecting to Typeform Data
Connecting to Typeform data looks just like connecting to any relational data source. Create a connection string using the required connection properties. For this article, you will pass the connection string as a parameter to the create_engine function.
Start by setting the Profile connection property to the location of the TypeForm Profile on disk (e.g. C:\profiles\TypeForm.apip). Next, set the ProfileSettings connection property to the connection string for TypeForm (see below).
TypeForm API Profile Settings
Authentication to TypeForm uses the OAuth standard.
To authenticate to TypeForm, you must first register and configure an OAuth application with TypeForm here: https://admin.typeform.com/account#/section/tokens. Your app will be assigned a client ID and a client secret which can be set in the connection string. More information on setting up an OAuth application can be found at https://developer.typeform.com/get-started/.
Note that there are several different use scenarios which all require different redirect URIs:
- CData Desktop Applications: CData desktop applications (Sync, API Server, ArcESB) accept OAuth tokens at /src/oauthCallback.rst. The host and port is the same as the default port used by the application. For example, if you use http://localhost:8019/ to access CData Sync then the redirect URI will be http://localhost:8019/src/oauthCallback.rst.
- CData Cloud Applications: CData cloud applications are similar to their desktop counterparts. If you access Connect Cloud at https://1.2.3.4/ then you should use the redirect https://1.2.3.4/src/oauthCallback.rst.
- Desktop Application: When using a desktop application, the URI https://localhost:33333 is recommended.
- Web Application: When developing a web application using the driver, use your own URI here such as https://my-website.com/oauth.
After setting the following connection properties, you are ready to connect:
- AuthScheme: Set this to OAuth.
- InitiateOAuth: Set this to GETANDREFRESH. You can use InitiateOAuth to manage the process to obtain the OAuthAccessToken.
- OAuthClientId: Set this to the Client Id that is specified in your app settings.
- OAuthClientSecret: Set this to Client Secret that is specified in your app settings.
- CallbackURL: Set this to the Redirect URI you specified in your app settings.
After installing the CData Typeform Connector, follow the procedure below to install the other required modules and start accessing Typeform through Python objects.
Install Required Modules
Use the pip utility to install the required modules and frameworks:
pip install pandas pip install dash pip install dash-daq
Visualize Typeform Data in Python
Once the required modules and frameworks are installed, we are ready to build our web app. Code snippets follow, but the full source code is available at the end of the article.
First, be sure to import the modules (including the CData Connector) with the following:
import os import dash import dash_core_components as dcc import dash_html_components as html import pandas as pd import cdata.api as mod import plotly.graph_objs as go
You can now connect with a connection string. Use the connect function for the CData Typeform Connector to create a connection for working with Typeform data.
cnxn = mod.connect("Profile=C:\profiles\TypeForm.apip;Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;InitiateOAuth=GETANDREFRESH;OAuthSettingsLocation=/PATH/TO/OAuthSettings.txt")")
Execute SQL to Typeform
Use the read_sql function from pandas to execute any SQL statement and store the result set in a DataFrame.
df = pd.read_sql("SELECT Id, Title FROM Tags WHERE SettingsIsPublic = 'true'", cnxn)
Configure the Web App
With the query results stored in a DataFrame, we can begin configuring the web app, assigning a name, stylesheet, and title.
app_name = 'dash-apiedataplot' external_stylesheets = ['https://codepen.io/chriddyp/pen/bWLwgP.css'] app = dash.Dash(__name__, external_stylesheets=external_stylesheets) app.title = 'CData + Dash'
Configure the Layout
The next step is to create a bar graph based on our Typeform data and configure the app layout.
trace = go.Bar(x=df.Id, y=df.Title, name='Id') app.layout = html.Div(children=[html.H1("CData Extension + Dash", style={'textAlign': 'center'}), dcc.Graph( id='example-graph', figure={ 'data': [trace], 'layout': go.Layout(title='Typeform Tags Data', barmode='stack') }) ], className="container")
Set the App to Run
With the connection, app, and layout configured, we are ready to run the app. The last lines of Python code follow.
if __name__ == '__main__': app.run_server(debug=True)
Now, use Python to run the web app and a browser to view the Typeform data.
python api-dash.py
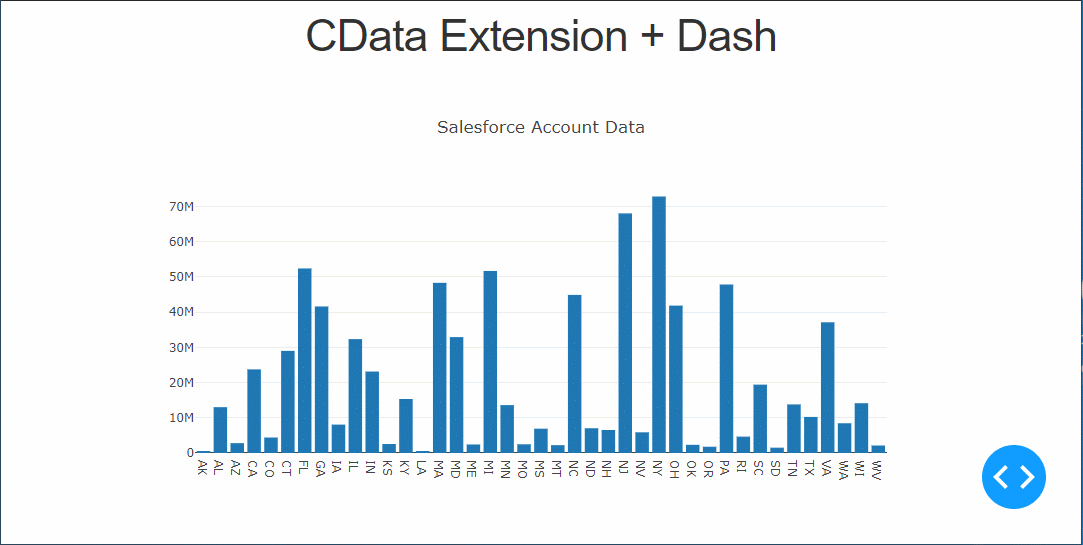
Free Trial & More Information
Download a free, 30-day trial of the CData API Driver for Python to start building Python apps with connectivity to Typeform data. Reach out to our Support Team if you have any questions.
Full Source Code
import os import dash import dash_core_components as dcc import dash_html_components as html import pandas as pd import cdata.api as mod import plotly.graph_objs as go cnxn = mod.connect("Profile=C:\profiles\TypeForm.apip;Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;InitiateOAuth=GETANDREFRESH;OAuthSettingsLocation=/PATH/TO/OAuthSettings.txt") df = pd.read_sql("SELECT Id, Title FROM Tags WHERE SettingsIsPublic = 'true'", cnxn) app_name = 'dash-apidataplot' external_stylesheets = ['https://codepen.io/chriddyp/pen/bWLwgP.css'] app = dash.Dash(__name__, external_stylesheets=external_stylesheets) app.title = 'CData + Dash' trace = go.Bar(x=df.Id, y=df.Title, name='Id') app.layout = html.Div(children=[html.H1("CData Extension + Dash", style={'textAlign': 'center'}), dcc.Graph( id='example-graph', figure={ 'data': [trace], 'layout': go.Layout(title='Typeform Tags Data', barmode='stack') }) ], className="container") if __name__ == '__main__': app.run_server(debug=True)