Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Connect to Sage 300 Data from Blazor Apps
Build ASP.NET Core Blazor C# apps that integrate with real-time Sage 300 data using standard SQL.
Blazor is a framework for developing modern, client-side web UIs using .NET technology. Instead of coding in JavaScript, developers can use the familiar C# language and .NET libraries to build app UIs.
The CData ADO.NET Provider for Sage 300 can be used with standard ADO.NET interfaces, such as LINQ and Entity Framework, to interact with live Sage 300 data. Since Blazor supports .NET Core, developers can use CData ADO.NET Providers in Blazor apps. In this article, we will guide you to build a simple Blazor app that talks to Sage 300 using standard SQL queries.
Install the CData ADO.NET Provider for Sage 300
CData ADO.NET Providers allow users to access Sage 300 just like they would access SQL Server, using simple SQL queries.
Install the Sage 300 ADO.NET Data Provider from the CData website or from NuGet. Search NuGet for "Sage 300 ADO.NET Data Provider."
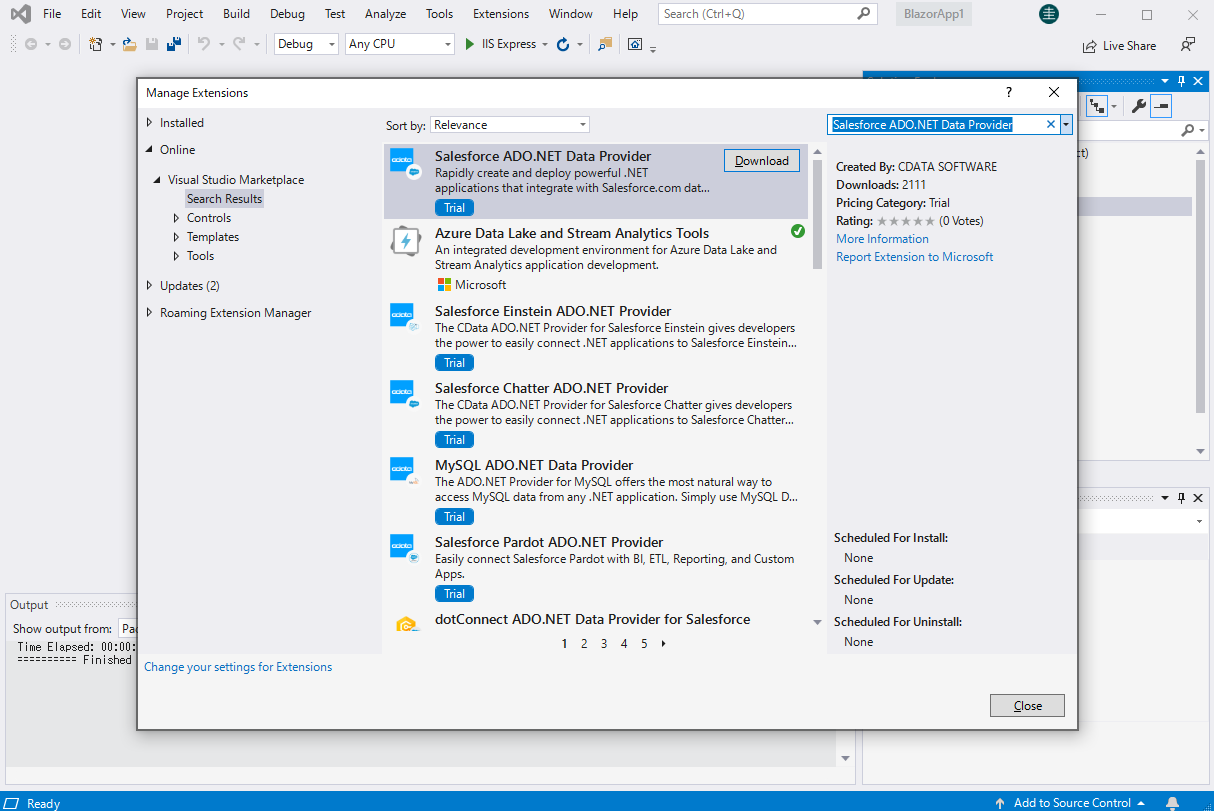
Create a Sage 300-Connected Blazor App
Start by creating a Blazor project that references the CData ADO.NET Provider for Sage 300
- Create a Blazor project on Visual Studio.
- From the Solution Explorer, right click Dependencies, then click Add Project Reference.
- In the Reference Manager, click the Browse button, and choose the .dll file of the installed ADO.NET Provider (e.g. System.Data.CData.Sage300.dll, typically located at C:\Program Files\CData\CData ADO.NET Provider for Sage 300\lib etstandard2.0).
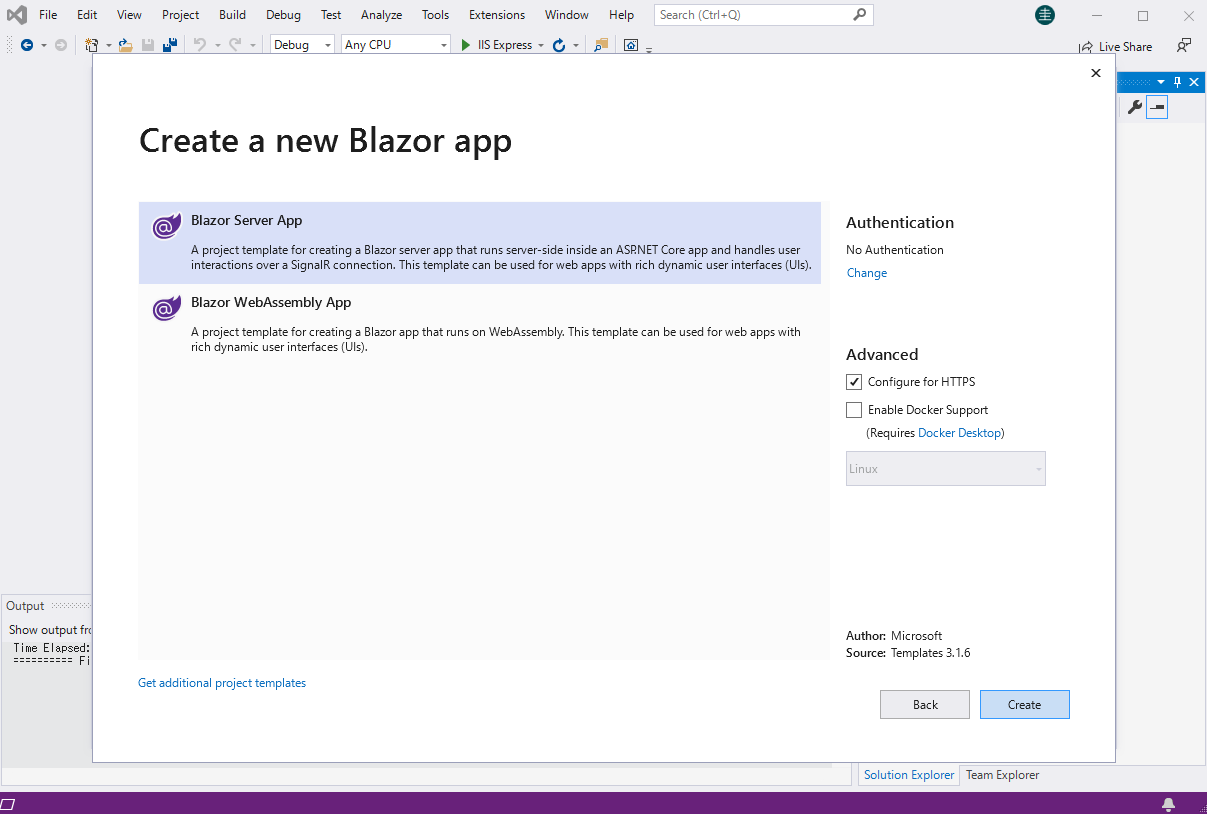
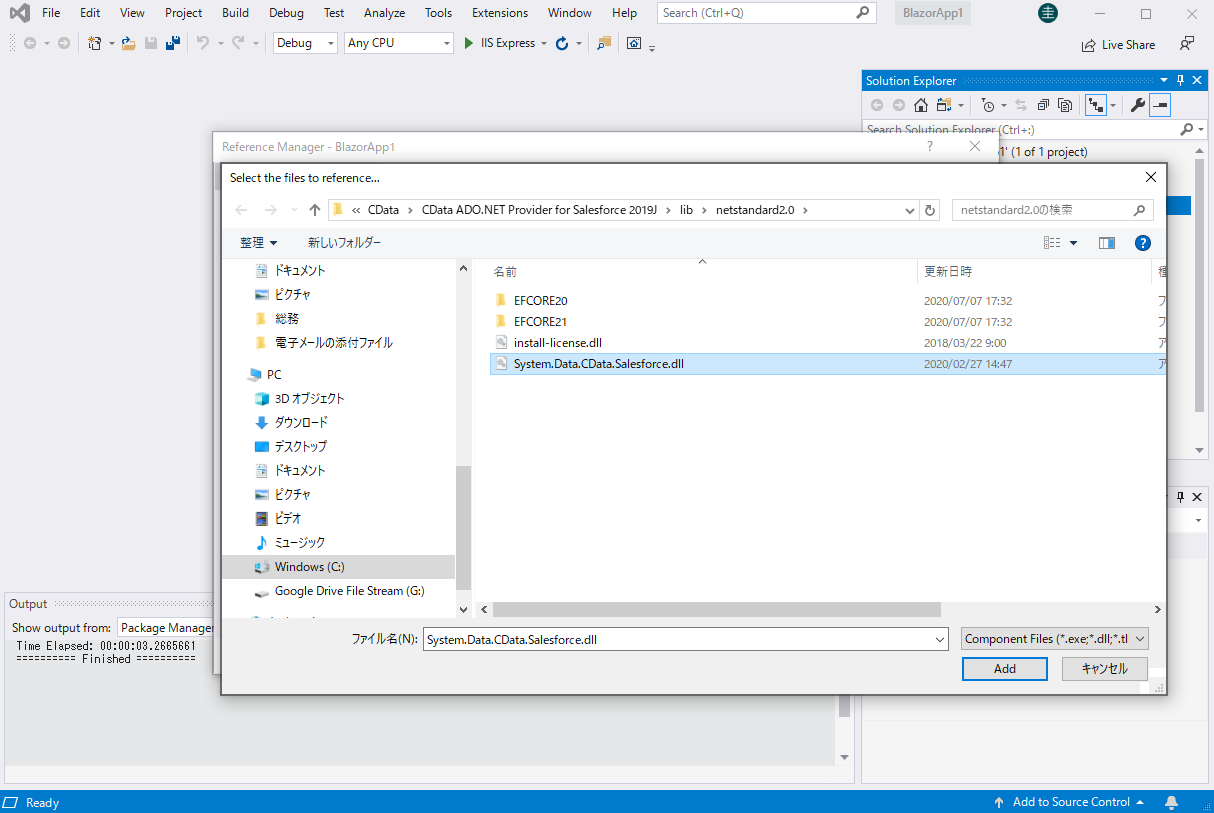
SELECT Sage 300 Data from the Blazor App
- Open the Index.razor file from the Project page.
- In a Sage300Connection object, set the connection string:
Sage 300 requires some initial setup in order to communicate over the Sage 300 Web API.
- Set up the security groups for the Sage 300 user. Give the Sage 300 user access to the
option under Security Groups (per each module required). - Edit both web.config files in the /Online/Web and /Online/WebApi folders; change the key AllowWebApiAccessForAdmin to true. Restart the webAPI app-pool for the settings to take.
- Once the user access is configured, click https://server/Sage300WebApi/ to ensure access to the web API.
Authenticate to Sage 300 using Basic authentication.
Connect Using Basic Authentication
You must provide values for the following properties to successfully authenticate to Sage 300. Note that the provider reuses the session opened by Sage 300 using cookies. This means that your credentials are used only on the first request to open the session. After that, cookies returned from Sage 300 are used for authentication.
- Url: Set this to the url of the server hosting Sage 300. Construct a URL for the Sage 300 Web API as follows: {protocol}://{host-application-path}/v{version}/{tenant}/ For example, http://localhost/Sage300WebApi/v1.0/-/.
- User: Set this to the username of your account.
- Password: Set this to the password of your account.
For example: User=SAMPLE;Password=password;URL=http://127.0.0.1/Sage300WebApi/v1/-/;Company=SAMINC;
- Set up the security groups for the Sage 300 user. Give the Sage 300 user access to the
- The code below creates a simple Blazor app for displaying Sage 300 data, using standard SQL to query Sage 300 just like SQL Server.
@page "/" @using System.Data; @using System.Data.CData.Sage300; <h1>Hello, world!</h1> Welcome to your Data app. <div class="row"> <div class="col-12"> @using (Sage300Connection connection = new Sage300Connection( "User=SAMPLE;Password=password;URL=http://127.0.0.1/Sage300WebApi/v1/-/;Company=SAMINC;")) { var sql = "SELECT InvoiceUniquifier, ApprovedLimit FROM OEInvoices WHERE AllowPartialShipments = 'Yes'"; var results = new DataTable(); Sage300DataAdapter dataAdapter = new Sage300DataAdapter(sql, connection); dataAdapter.Fill(results); <table class="table table-bordered"> <thead class="thead-light"> <tr> @foreach (DataColumn item in results.Rows[0].Table.Columns) { <th scope="col">@item.ColumnName</th> } </tr> </thead> <tbody> @foreach (DataRow row in results.Rows) { <tr> @foreach (var column in row.ItemArray) { <td>@column.ToString()</td> } </tr> } </tbody> </table> } </div> </div>
- Rebuild and run the project. The ADO.NET Provider renders Sage 300 data as an HTML table in the Blazor app.
At this point, you have a Sage 300-connected Blazor app, capable of working with live Sage 300 data just like you would work with a SQL Server instance. Download a free, 30-day trial and start working with live Sage 300 data in your Blazor apps today.