Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create a Data Access Object for Klipfolio Data using JDBI
A brief overview of creating a SQL Object API for Klipfolio data in JDBI.
JDBI is a SQL convenience library for Java that exposes two different style APIs, a fluent style and a SQL object style. The CData JDBC Driver for Klipfolio integrates connectivity to live Klipfolio data in Java applications. By pairing these technologies, you gain simple, programmatic access to Klipfolio data. This article walks through building a basic Data Access Object (DAO) and the accompanying code to read Klipfolio data.
Create a DAO for the Klipfolio DataSources Entity
The interface below declares the desired behavior for the SQL object to create a single method for each SQL statement to be implemented.
public interface MyDataSourcesDAO {
//request specific data from Klipfolio (String type is used for simplicity)
@SqlQuery("SELECT Name FROM DataSources WHERE IsDynamic = :isDynamic")
String findNameByIsDynamic(@Bind("isDynamic") String isDynamic);
/*
* close with no args is used to close the connection
*/
void close();
}
Open a Connection to Klipfolio
Collect the necessary connection properties and construct the appropriate JDBC URL for connecting to Klipfolio.
Start by setting the Profile connection property to the location of the Klipfolio Profile on disk (e.g. C:\profiles\Klipfolio.apip). Next, set the ProfileSettings connection property to the connection string for Klipfolio (see below).
Klipfolio API Profile Settings
In order to authenticate to Klipfolio, you'll need to provide your API Key. You can generate an API key from the Klipfolio Dashboard app through either the My Profile page or from Users if you are an administrator (you must have the user.manage permission). Set the API Key in the ProfileSettings property to connect.
Built-in Connection String Designer
For assistance in constructing the JDBC URL, use the connection string designer built into the Klipfolio JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.api.jar
Fill in the connection properties and copy the connection string to the clipboard.
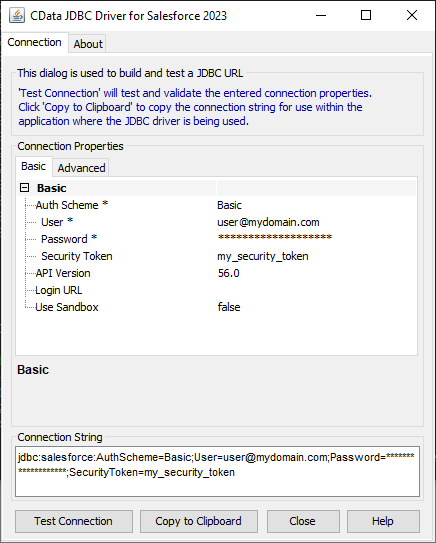
A connection string for Klipfolio will typically look like the following:
jdbc:api:Profile=C:\profiles\Klipfolio.apip;ProfileSettings='APIKey=your_api_key';
Use the configured JDBC URL to obtain an instance of the DAO interface. The particular method shown below will open a handle bound to the instance, so the instance needs to be closed explicitly to release the handle and the bound JDBC connection.
DBI dbi = new DBI("jdbc:api:Profile=C:\profiles\Klipfolio.apip;ProfileSettings='APIKey=your_api_key';");
MyDataSourcesDAO dao = dbi.open(MyDataSourcesDAO.class);
//do stuff with the DAO
dao.close();
Read Klipfolio Data
With the connection open to Klipfolio, simply call the previously defined method to retrieve data from the DataSources entity in Klipfolio.
//disply the result of our 'find' method
String name = dao.findNameByIsDynamic("true");
System.out.println(name);
Since the JDBI library is able to work with JDBC connections, you can easily produce a SQL Object API for Klipfolio by integrating with the CData JDBC Driver for Klipfolio. Download a free trial and work with live Klipfolio data in custom Java applications today.