Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →DataBind JotForm Data to the DevExpress Data Grid
Use the CData ADO.NET Provider for JotForm with the DevExpress Windows Forms and Web controls to provide JotForm data to a chart.
The ADO.NET Provider for JotForm by CData incorporates conventional ADO.NET data access components compatible with third-party controls. You can adhere to the standard ADO.NET data binding procedures to establish two-way access to real-time data through UI controls. This article will demonstrate the utilization of CData components for data binding with DevExpress UI Controls (Windows Forms and Web controls), specifically binding to a chart that visualizes live data.
Start by setting the Profile connection property to the location of the JotForm Profile on disk (e.g. C:\profiles\JotForm.apip). Next, set the ProfileSettings connection property to the connection string for JotForm (see below).
JotForm API Profile Settings
You will need to find your JotForm API Key in order to authenticate. To obtain an API Key, go to 'My Account' > 'API Section' > 'Create a New API Key'. Once you've created your new API Key, you can set it in the ProfileSettings connection property.
Custom Enterprise API Domains
Enterprise customers of JotForm are given custom API domains to connect to, rather than the default 'api.jotform.com' domain. If you are an enterprise JotForm customer, then set Domain to you custom API hostname, such as 'your-domain.com' or 'subdomain.jotform.com', inside the ProfileSettings connection property. Conversely, if you do not have a custom domain and still need to connect to 'api.jotform.com', then leave Domain undefined and set only APIKey.
Windows Forms Controls
The code below shows how to populate a DevExpress chart with JotForm data. The APIDataAdapter binds to the Series property of the chart control. The Diagram property of the control defines the x- and y-axes as the column names.
using (APIConnection connection = new APIConnection(
"Profile=C:\profiles\JotForm.apip;ProfileSettings='APIKey=my_api_key;Domain=my_custom_domain';")) {
APIDataAdapter dataAdapter = new APIDataAdapter(
"SELECT Id, Title FROM Forms WHERE Status = 'ENABLED'", connection);
DataTable table = new DataTable();
dataAdapter.Fill(table);
DevExpress.XtraCharts.Series series = new DevExpress.XtraCharts.Series();
chartControl1.Series.Add(series);
series.DataSource = table;
series.ValueDataMembers.AddRange(new string[] { "Title" });
series.ArgumentScaleType = DevExpress.XtraCharts.ScaleType.Qualitative;
series.ArgumentDataMember = "Id";
series.ValueScaleType = DevExpress.XtraCharts.ScaleType.Numerical;
chartControl1.Legend.Visibility = DevExpress.Utils.DefaultBoolean.False;
((DevExpress.XtraCharts.SideBySideBarSeriesView)series.View).ColorEach = true;
}
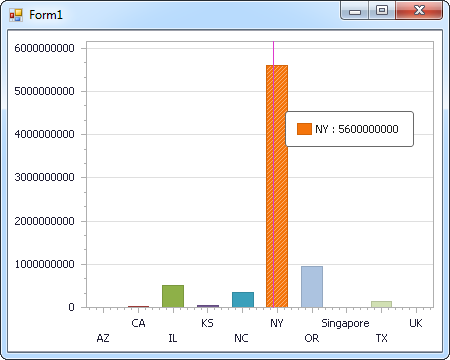
Web Controls
The code below shows how to populate a DevExpress Web control with JotForm data. The APIDataAdapter binds to the Series property of the chart; the Diagram property defines the x- and y-axes as the column names.
using DevExpress.XtraCharts;
using (APIConnection connection = new APIConnection(
"Profile=C:\profiles\JotForm.apip;ProfileSettings='APIKey=my_api_key;Domain=my_custom_domain';"))
{
APIDataAdapter APIDataAdapter1 = new APIDataAdapter("SELECT Id, Title FROM Forms WHERE Status = 'ENABLED'", connection);
DataTable table = new DataTable();
APIDataAdapter1.Fill(table);
DevExpress.XtraCharts.Series series = new Series("Series1", ViewType.Bar);
WebChartControl1.Series.Add(series);
series.DataSource = table;
series.ValueDataMembers.AddRange(new string[] { "Title" });
series.ArgumentScaleType = ScaleType.Qualitative;
series.ArgumentDataMember = "Id";
series.ValueScaleType = ScaleType.Numerical;
((DevExpress.XtraCharts.SideBySideBarSeriesView)series.View).ColorEach = true;
}
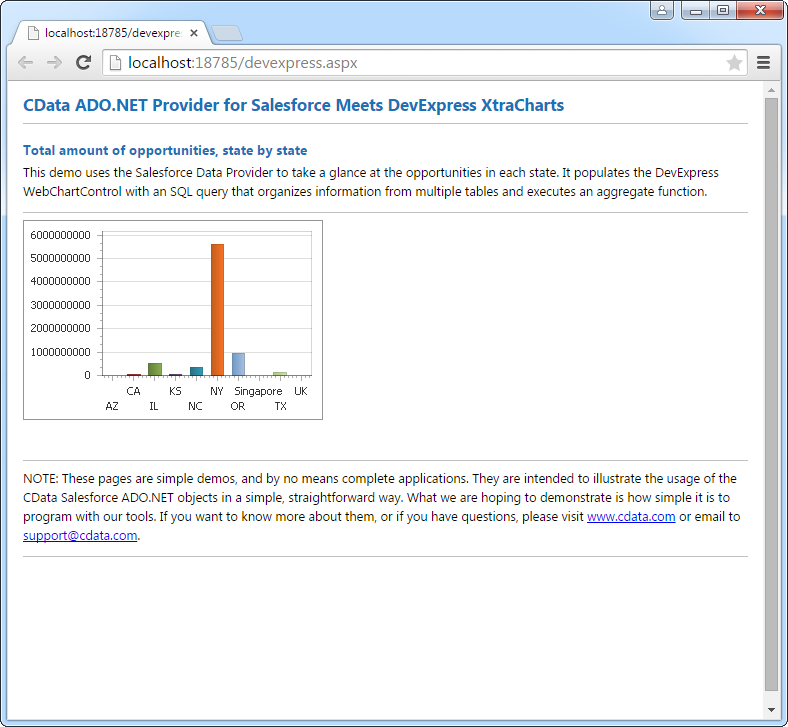