Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Connect to Harvest Data from Blazor Apps
Build ASP.NET Core Blazor C# apps that integrate with real-time Harvest data using standard SQL.
Blazor is a framework for developing modern, client-side web UIs using .NET technology. Instead of coding in JavaScript, developers can use the familiar C# language and .NET libraries to build app UIs.
The CData API Driver for ADO.NET can be used with standard ADO.NET interfaces, such as LINQ and Entity Framework, to interact with live Harvest data. Since Blazor supports .NET Core, developers can use CData ADO.NET Providers in Blazor apps. In this article, we will guide you to build a simple Blazor app that talks to Harvest using standard SQL queries.
Install the CData API Driver for ADO.NET
CData ADO.NET Providers allow users to access Harvest just like they would access SQL Server, using simple SQL queries.
Install the Harvest ADO.NET Data Provider from the CData website or from NuGet. Search NuGet for "Harvest ADO.NET Data Provider."
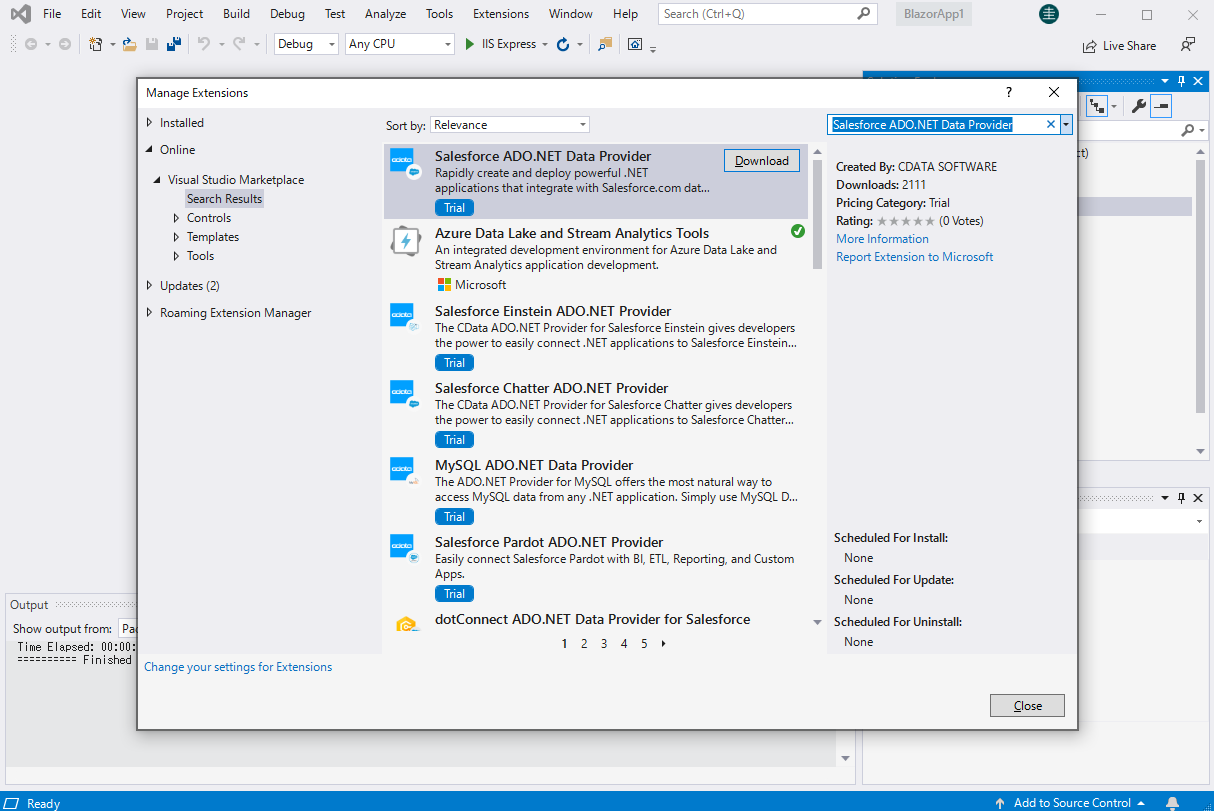
Create a Harvest-Connected Blazor App
Start by creating a Blazor project that references the CData API Driver for ADO.NET
- Create a Blazor project on Visual Studio.
- From the Solution Explorer, right click Dependencies, then click Add Project Reference.
- In the Reference Manager, click the Browse button, and choose the .dll file of the installed ADO.NET Provider (e.g. System.Data.CData.API.dll, typically located at C:\Program Files\CData\CData API Driver for ADO.NET\lib etstandard2.0).
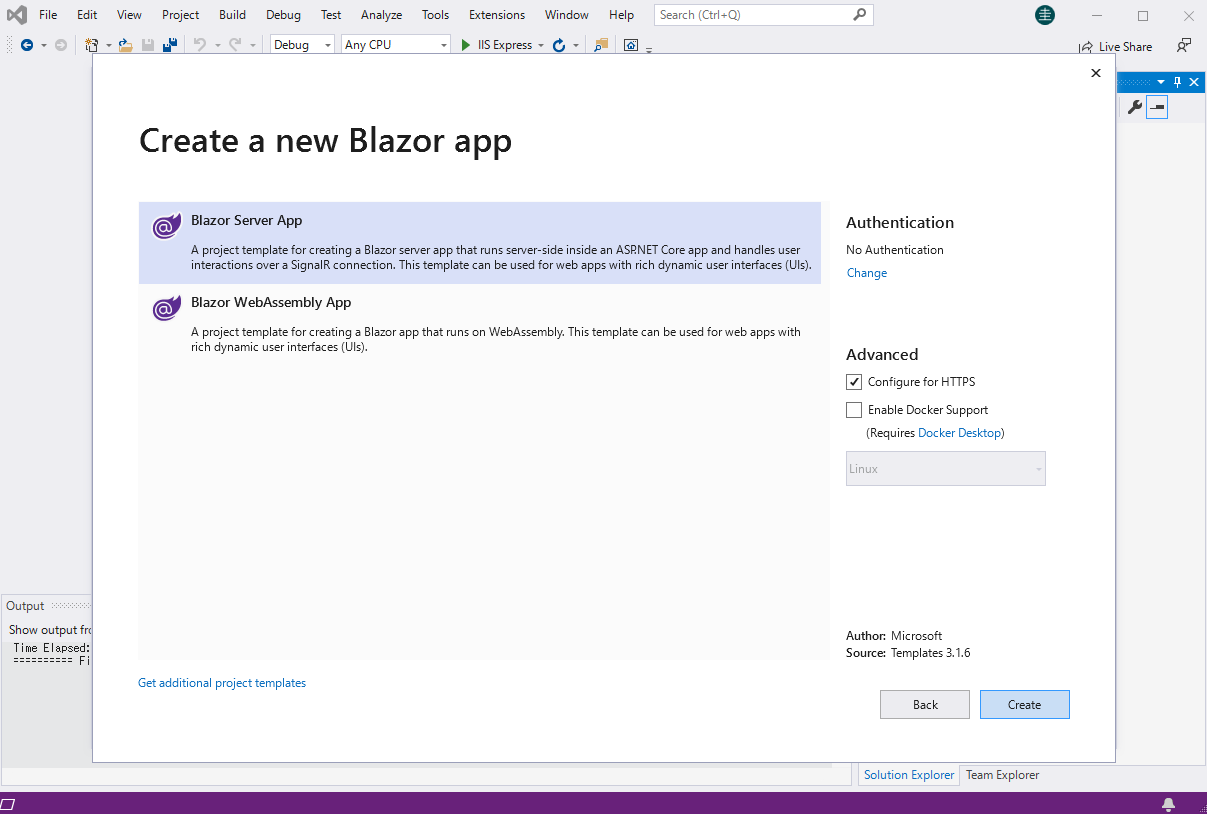
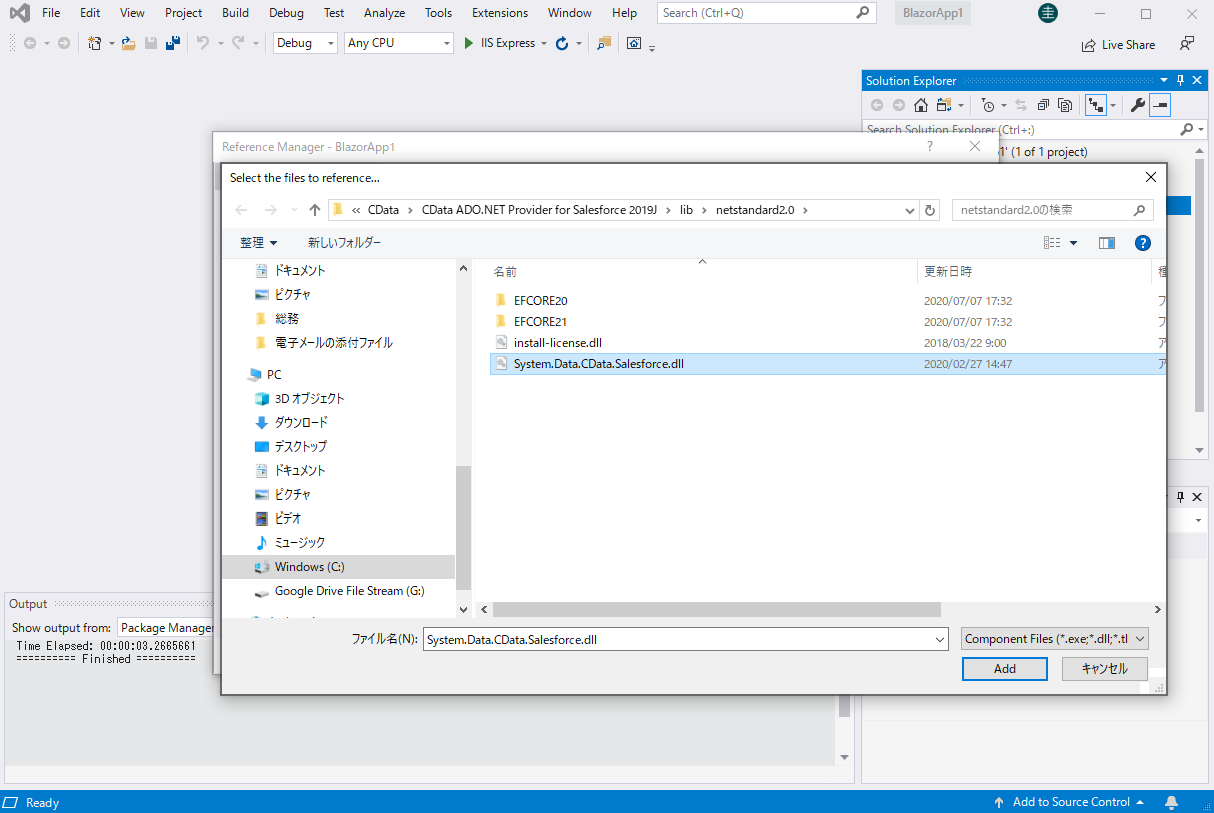
SELECT Harvest Data from the Blazor App
- Open the Index.razor file from the Project page.
- In a APIConnection object, set the connection string:
Start by setting the Profile connection property to the location of the Harvest Profile on disk (e.g. C:\profiles\Harvest.apip). Next, set the ProfileSettings connection property to the connection string for Harvest (see below).
Harvest API Profile Settings
To authenticate to Harvest, you can use either Token authentication or the OAuth standard. Use Basic authentication to connect to your own data. Use OAuth to allow other users to connect to their data.
Using Token Authentication
To use Token Authentication, set the APIKey to your Harvest Personal Access Token in the ProfileSettings connection property. In addition to APIKey, set your AccountId in ProfileSettings to connect.
Using OAuth Authentication
First, register an OAuth2 application with Harvest. The application can be created from the "Developers" section of Harvest ID.
After setting the following connection properties, you are ready to connect:
- ProfileSettings: Set your AccountId in ProfileSettings.
- AuthScheme: Set this to OAuth.
- OAuthClientId: Set this to the client ID that you specified in your app settings.
- OAuthClientSecret: Set this to the client secret that you specified in your app settings.
- CallbackURL: Set this to the Redirect URI that you specified in your app settings.
- InitiateOAuth: Set this to GETANDREFRESH. You can use InitiateOAuth to manage how the driver obtains and refreshes the OAuthAccessToken.
For example: Profile=C:\profiles\Harvest.apip;ProfileSettings='APIKey=my_personal_key;AccountId=_your_account_id';
- The code below creates a simple Blazor app for displaying Harvest data, using standard SQL to query Harvest just like SQL Server.
@page "/" @using System.Data; @using System.Data.CData.API; <h1>Hello, world!</h1> Welcome to your Data app. <div class="row"> <div class="col-12"> @using (APIConnection connection = new APIConnection( "Profile=C:\profiles\Harvest.apip;ProfileSettings='APIKey=my_personal_key;AccountId=_your_account_id';")) { var sql = "SELECT Id, ClientName FROM Invoices WHERE State = 'open'"; var results = new DataTable(); APIDataAdapter dataAdapter = new APIDataAdapter(sql, connection); dataAdapter.Fill(results); <table class="table table-bordered"> <thead class="thead-light"> <tr> @foreach (DataColumn item in results.Rows[0].Table.Columns) { <th scope="col">@item.ColumnName</th> } </tr> </thead> <tbody> @foreach (DataRow row in results.Rows) { <tr> @foreach (var column in row.ItemArray) { <td>@column.ToString()</td> } </tr> } </tbody> </table> } </div> </div>
- Rebuild and run the project. The ADO.NET Provider renders Harvest data as an HTML table in the Blazor app.
At this point, you have a Harvest-connected Blazor app, capable of working with live Harvest data just like you would work with a SQL Server instance. Download a free, 30-day trial and start working with live Harvest data in your Blazor apps today.