Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create Dynamic Cvent Grids Using the Infragistics XamDataGrid
Learn how you can connect Cvent to Infragistics XamDataGrid to build dynamic grids.
Using Infragistics WPF UI controls, you can build contemporary applications reminiscent of Microsoft Office for both desktop and touch-based devices. When coupled with the CData ADO.NET Provider for Cvent, you gain the capability to construct interactive grids, charts, and various other visual elements while directly accessing real-time data from Cvent data. This article will guide you through the process of creating a dynamic grid within Visual Studio using the Infragistics XamDataGrid control.
You will need to install the Infragistics WPF UI components to continue. Download a free trial here: https://www.infragistics.com/products/wpf.
Create a WPF Project
Open VisualStudio and create a new WPF project.
Add a TextBox for passing a SQL query to the CData ADO.NET Provider and a Button for executing the query.
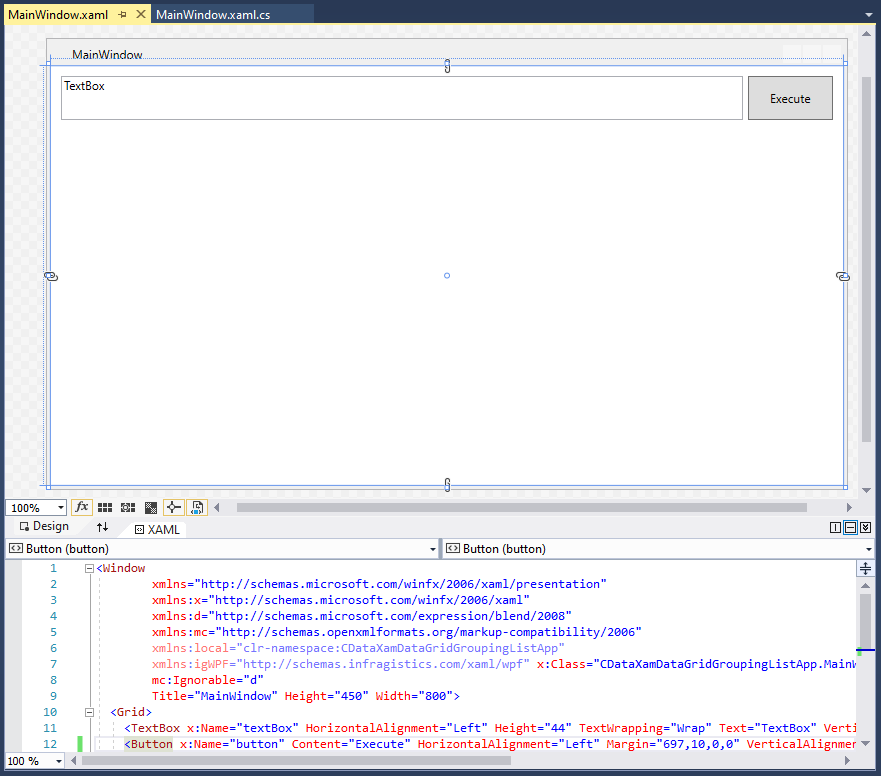
The XAML at this stage is as follows:
< Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:CDataXamDataGridGroupingListApp" xmlns:igWPF="http://schemas.infragistics.com/xaml/wpf" x:Class="CDataXamDataGridGroupingListApp.MainWindow" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800"> < Grid> < TextBox x:Name="textBox" HorizontalAlignment="Left" Height="44" TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top" Width="682" Margin="10,10,0,0"/> < Button x:Name="button" Content="Execute" HorizontalAlignment="Left" Margin="697,10,0,0" VerticalAlignment="Top" Width="85" Height="44"/> < /Grid> < /Window>
Add and Configure a XamDataGrid
After adding the initial controls, add a XamDataGrid to the App. The component will appear in the Visual Studio toolbox.
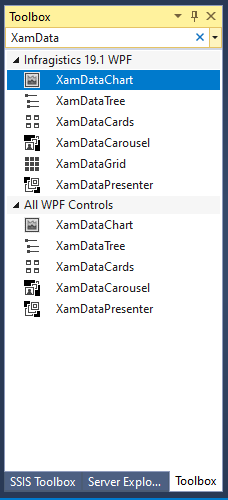
Arrange the component on the designer so that it is below the TextBox & Button and linked to the boundaries of the app.
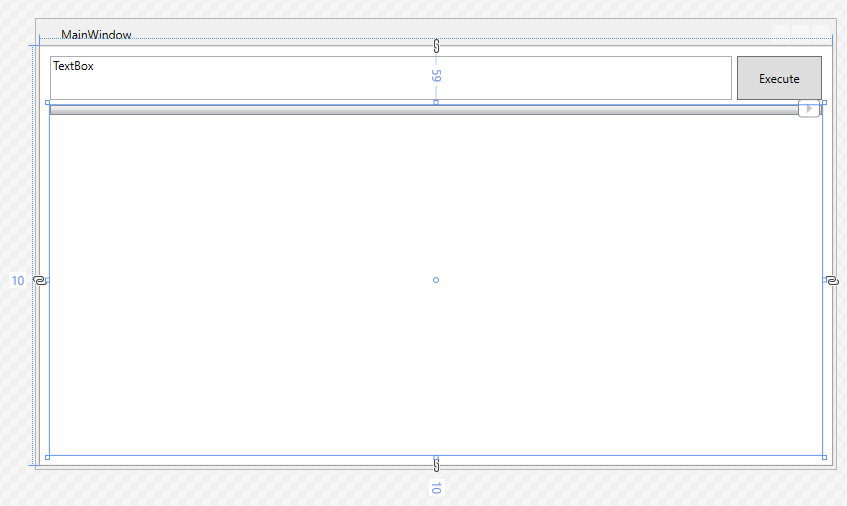
Once the XamDataGrid is placed, edit the XAML to set the XamDataGrid DataSource attribute to "{Binding}" and set the FieldSettings AllowRecordFiltering and AllowSummaries attributes to "true." Next, add an empty method as the Click event handler for the Button component. The XAML at this stage is as follows:
< Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:CDataXamDataGridGroupingListApp" xmlns:igWPF="http://schemas.infragistics.com/xaml/wpf" x:Class="CDataXamDataGridGroupingListApp.MainWindow" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800"> < Grid> < TextBox x:Name="textBox" HorizontalAlignment="Left" Height="44" TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top" Width="682" Margin="10,10,0,0"/> < Button x:Name="button" Content="Execute" HorizontalAlignment="Left" Margin="697,10,0,0" VerticalAlignment="Top" Width="85" Click="Button_Click" Height="44"/> < igWPF:XamDataGrid Margin="10,59,10,10" DataSource="{Binding}"> < igWPF:XamDataGrid.FieldSettings> < igWPF:FieldSettings AllowSummaries="True" AllowRecordFiltering="True"/> < /igWPF:XamDataGrid.FieldSettings> < /igWPF:XamDataGrid> < /Grid> < /Window>
Connect to and Query Cvent
The last step in building our WPG App with a dynamic DataGrid is connecting to and querying live Cvent data. First add a reference to the CData ADO.NET Provider to the project (typically found in C:\Program Files\CData[product_name]\lib).
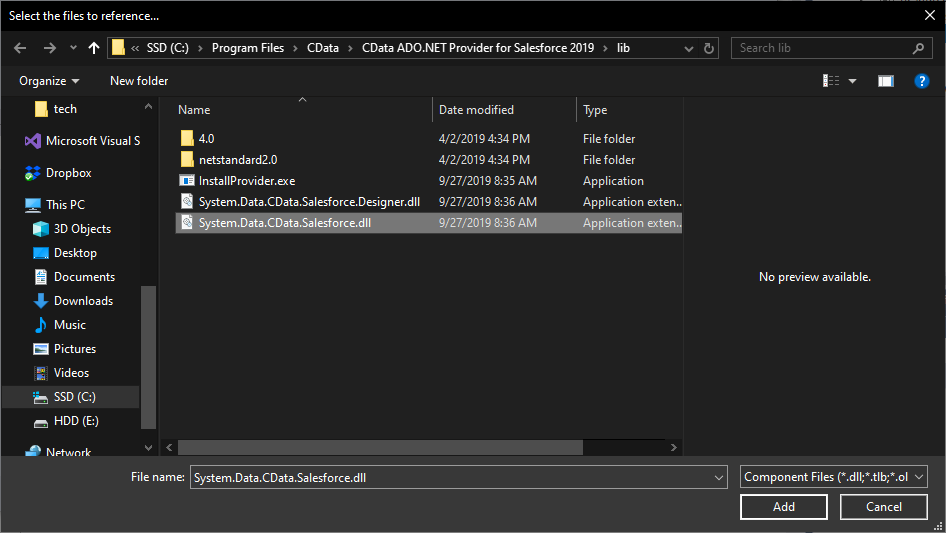
Next, add the Provider to the namespace, along with the standard Data library:
using System.Data.CData.Cvent; using System.Data;
Finally, add the code to connect to Cvent and query using the text from the TextBox to the Click event handler.
Before you can authenticate to Cvent, you must create a workspace and an OAuth application.
Creating a Workspace
To create a workspace:
- Sign into Cvent and navigate to App Switcher (the blue button in the upper right corner of the page) >> Admin.
- In the Admin menu, navigate to Integrations >> REST API.
- A new tab launches for Developer Management. Click on Manage API Access in the new tab.
- Create a Workspace and name it. Select the scopes you would like your developers to have access to. Scopes control what data domains the developer can access.
- Choose All to allow developers to choose any scope, and any future scopes added to the REST API.
- Choose Custom to limit the scopes developers can choose for their OAuth apps to selected scopes. To access all tables exposed by the driver, you need to set the following scopes:
event/attendees:read event/attendees:write event/contacts:read event/contacts:write event/custom-fields:read event/custom-fields:write event/events:read event/events:write event/sessions:delete event/sessions:read event/sessions:write event/speakers:delete event/speakers:read event/speakers:write budget/budget-items:read budget/budget-items:write exhibitor/exhibitors:read exhibitor/exhibitors:write survey/surveys:read survey/surveys:write
Creating an OAuth Application
After you have set up a Workspace and invited them, developers can sign up and create a custom OAuth app. See the Creating a Custom OAuth Application section in the Help documentation for more information.
Connecting to Cvent
After creating an OAuth application, set the following connection properties to connect to Cvent:
- InitiateOAuth: GETANDREFRESH. Used to automatically get and refresh the OAuthAccessToken.
- OAuthClientId: The Client ID associated with the OAuth application. You can find this on the Applications page in the Cvent Developer Portal.
- OAuthClientSecret: The Client secret associated with the OAuth application. You can find this on the Applications page in the Cvent Developer Portal.
private void Button_Click(object sender, RoutedEventArgs e) { //connecting to Cvent string connString = "OAuthClientId=MyOAuthClientId;OAuthClientSecret=MyOAuthClientSecret;"; using (var conn = new CventConnection(connString)) { //using the query from the TextBox var dataAdapter = new CventDataAdapter(textBox.Text, conn); var table = new DataTable(); dataAdapter.Fill(table); //passing the DataRowCollection to the DataContext // for use in the XamDataGrid this.DataContext = table.Rows; } }
Run the Application
With the app fully configured, we are ready to display Cvent data in our XamDataGrid. When you click "Execute," the app connects to Cvent and submits the SQL query through the CData ADO.NET Provider.
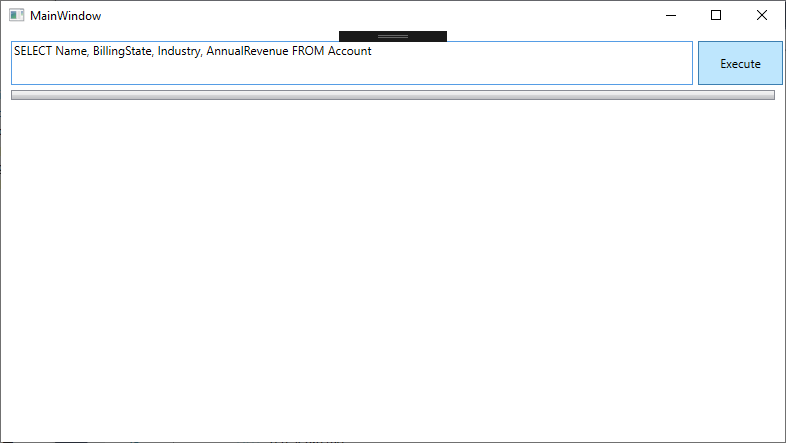
Live Cvent data is displayed in the grid.
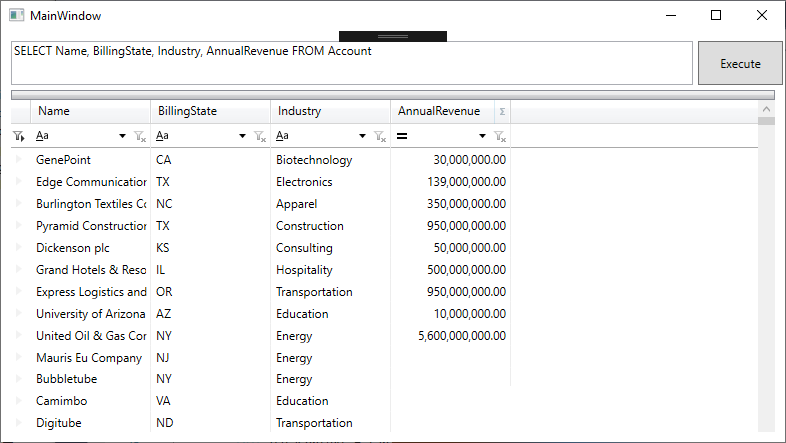
Group the data by dragging and dropping a column name into the header.
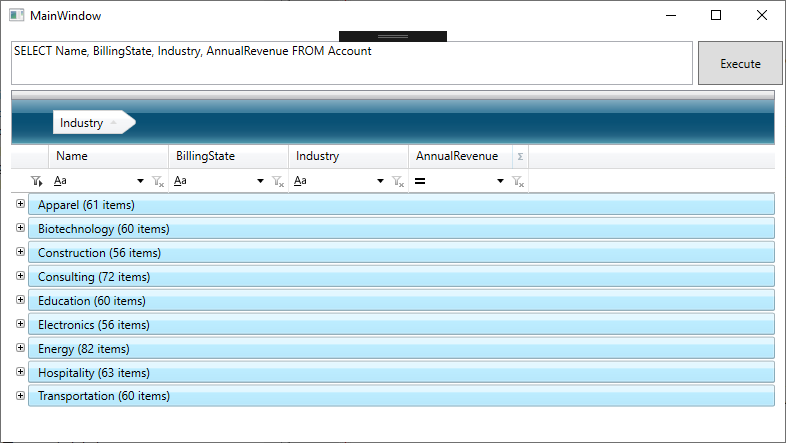
As you add groupings and filters, the underlying SQL query is submitted directly to Cvent, making it possible to drill down into live Cvent data to find only the specific information you need.
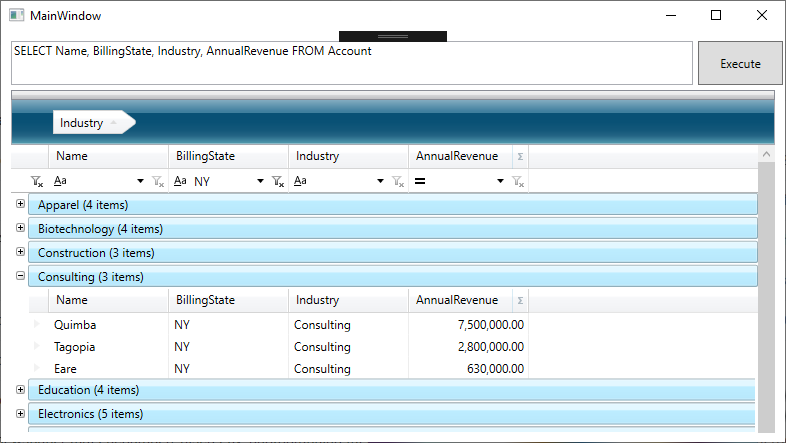
Free Trial & More Information
At this point, you have created a dynamic WPF App with access to live Cvent data. For more information, visit the CData ADO.NET Provider page. Download a free, 30-day trial and start working live Cvent data in apps built using the Infragistics UI controls today.