Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create a Data Access Object for Bullhorn CRM Data using JDBI
A brief overview of creating a SQL Object API for Bullhorn CRM data in JDBI.
JDBI is a SQL convenience library for Java that exposes two different style APIs, a fluent style and a SQL object style. The CData JDBC Driver for Bullhorn CRM integrates connectivity to live Bullhorn CRM data in Java applications. By pairing these technologies, you gain simple, programmatic access to Bullhorn CRM data. This article walks through building a basic Data Access Object (DAO) and the accompanying code to read and write Bullhorn CRM data.
Create a DAO for the Bullhorn CRM Candidate Entity
The interface below declares the desired behavior for the SQL object to create a single method for each SQL statement to be implemented.
public interface MyCandidateDAO {
//insert new data into Bullhorn CRM
@SqlUpdate("INSERT INTO Candidate (CandidateName, CandidateName) values (:candidateName, :candidateName)")
void insert(@Bind("candidateName") String candidateName, @Bind("candidateName") String candidateName);
//request specific data from Bullhorn CRM (String type is used for simplicity)
@SqlQuery("SELECT CandidateName FROM Candidate WHERE CandidateName = :candidateName")
String findCandidateNameByCandidateName(@Bind("candidateName") String candidateName);
/*
* close with no args is used to close the connection
*/
void close();
}
Open a Connection to Bullhorn CRM
Collect the necessary connection properties and construct the appropriate JDBC URL for connecting to Bullhorn CRM.
Begin by providing your Bullhorn CRM account credentials in the following:
- DataCenterCode: Set this to the data center code which responds to your data center. Refer to the list of data-center-specific Bullhorn API URLs: https://bullhorn.github.io/Data-Center-URLs/
If you are uncertain about your data center code, codes like CLS2, CLS21, etc. are cluster IDs that are contained in a user's browser URL (address bar) once they are logged in.
Example: https://cls21.bullhornstaffing.com/BullhornSTAFFING/MainFrame.jsp?#no-ba... indicates that the logged in user is on CLS21.
Authenticating with OAuth
Bullhorn CRM uses the OAuth 2.0 authentication standard. To authenticate using OAuth, create and configure a custom OAuth app. See the Help documentation for more information.
Built-in Connection String Designer
For assistance in constructing the JDBC URL, use the connection string designer built into the Bullhorn CRM JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.bullhorncrm.jar
Fill in the connection properties and copy the connection string to the clipboard.
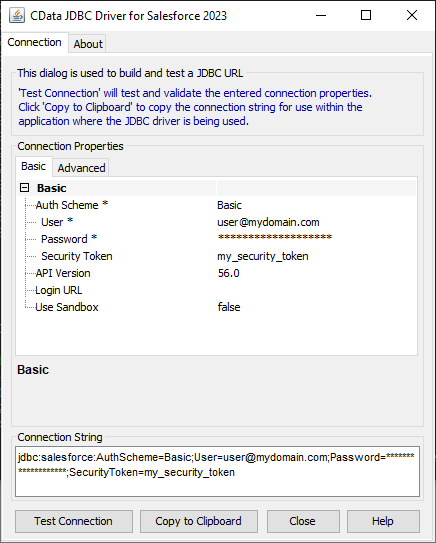
A connection string for Bullhorn CRM will typically look like the following:
jdbc:bullhorncrm:DataCenterCode=CLS33;OAuthClientId=myoauthclientid;OAuthClientSecret=myoauthclientsecret;InitiateOAuth=GETANDREFRESH
Use the configured JDBC URL to obtain an instance of the DAO interface. The particular method shown below will open a handle bound to the instance, so the instance needs to be closed explicitly to release the handle and the bound JDBC connection.
DBI dbi = new DBI("jdbc:bullhorncrm:DataCenterCode=CLS33;OAuthClientId=myoauthclientid;OAuthClientSecret=myoauthclientsecret;InitiateOAuth=GETANDREFRESH");
MyCandidateDAO dao = dbi.open(MyCandidateDAO.class);
//do stuff with the DAO
dao.close();
Read Bullhorn CRM Data
With the connection open to Bullhorn CRM, simply call the previously defined method to retrieve data from the Candidate entity in Bullhorn CRM.
//disply the result of our 'find' method
String candidateName = dao.findCandidateNameByCandidateName("Jane Doe");
System.out.println(candidateName);
Write Bullhorn CRM Data
It is also simple to write data to Bullhorn CRM, using the previously defined method.
//add a new entry to the Candidate entity
dao.insert(newCandidateName, newCandidateName);
Since the JDBI library is able to work with JDBC connections, you can easily produce a SQL Object API for Bullhorn CRM by integrating with the CData JDBC Driver for Bullhorn CRM. Download a free trial and work with live Bullhorn CRM data in custom Java applications today.