Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Connect to BigCommerce Data from Blazor Apps
Build ASP.NET Core Blazor C# apps that integrate with real-time BigCommerce data using standard SQL.
Blazor is a framework for developing modern, client-side web UIs using .NET technology. Instead of coding in JavaScript, developers can use the familiar C# language and .NET libraries to build app UIs.
The CData ADO.NET Provider for BigCommerce can be used with standard ADO.NET interfaces, such as LINQ and Entity Framework, to interact with live BigCommerce data. Since Blazor supports .NET Core, developers can use CData ADO.NET Providers in Blazor apps. In this article, we will guide you to build a simple Blazor app that talks to BigCommerce using standard SQL queries.
Install the CData ADO.NET Provider for BigCommerce
CData ADO.NET Providers allow users to access BigCommerce just like they would access SQL Server, using simple SQL queries.
Install the BigCommerce ADO.NET Data Provider from the CData website or from NuGet. Search NuGet for "BigCommerce ADO.NET Data Provider."
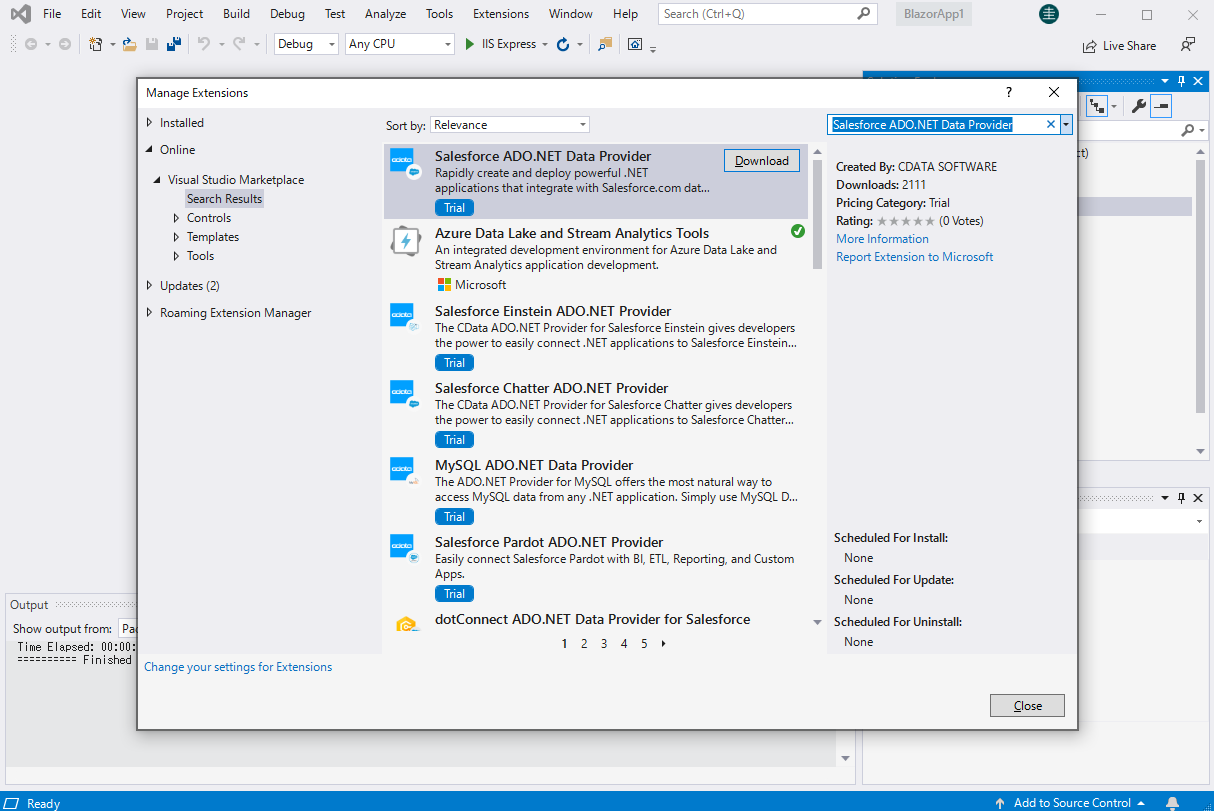
Create a BigCommerce-Connected Blazor App
Start by creating a Blazor project that references the CData ADO.NET Provider for BigCommerce
- Create a Blazor project on Visual Studio.
- From the Solution Explorer, right click Dependencies, then click Add Project Reference.
- In the Reference Manager, click the Browse button, and choose the .dll file of the installed ADO.NET Provider (e.g. System.Data.CData.BigCommerce.dll, typically located at C:\Program Files\CData\CData ADO.NET Provider for BigCommerce\lib etstandard2.0).
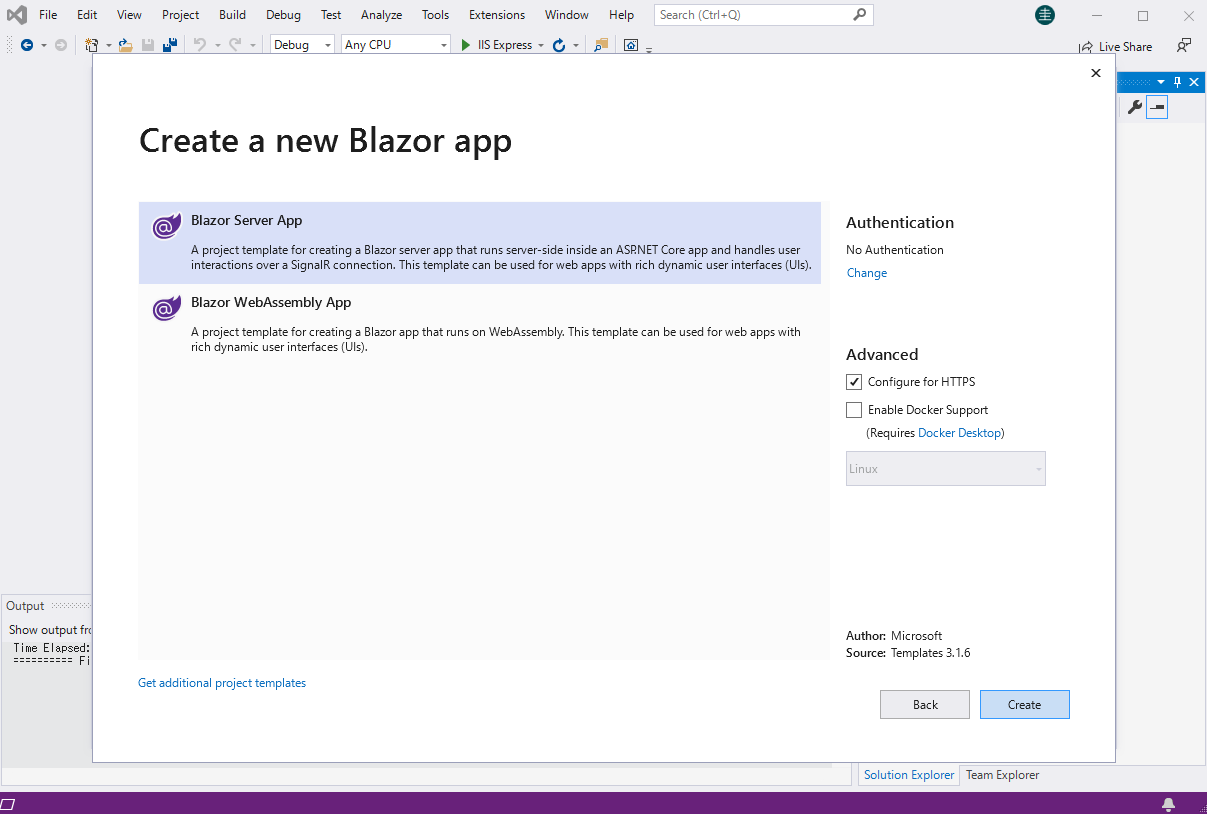
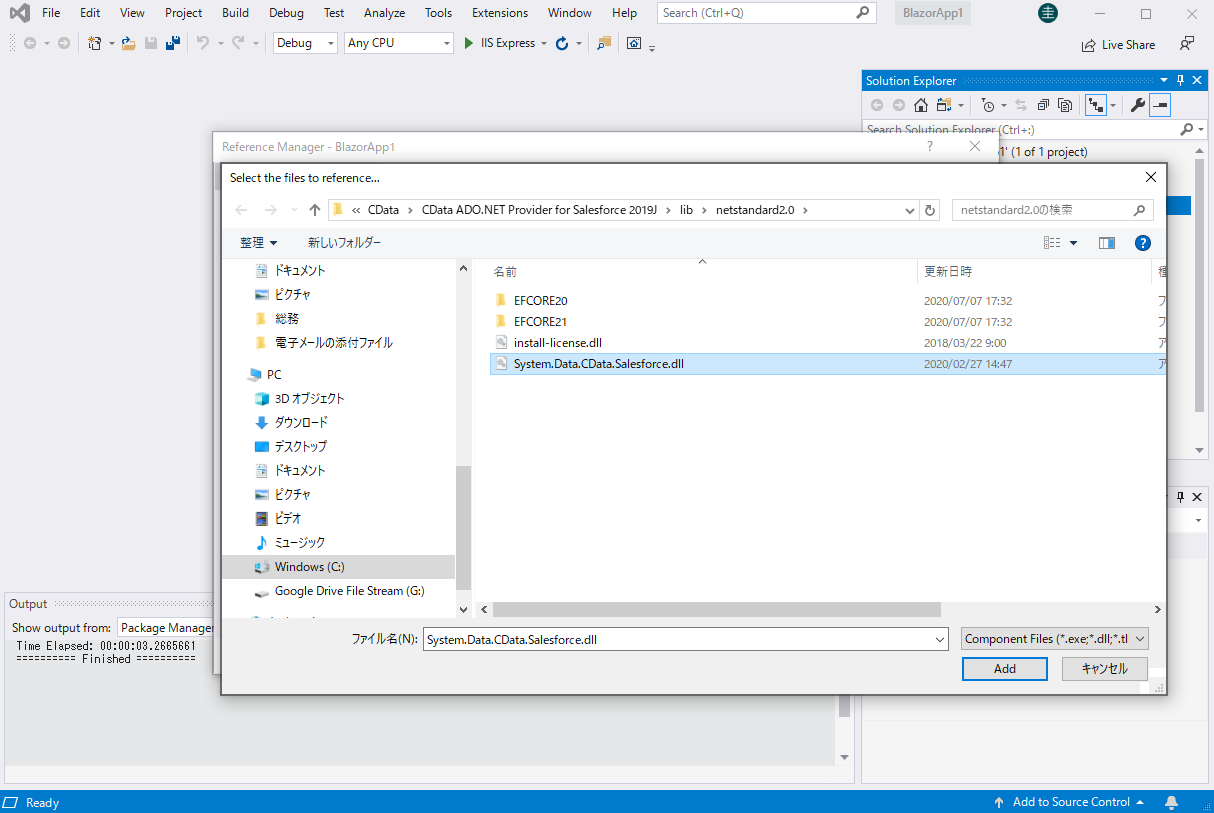
SELECT BigCommerce Data from the Blazor App
- Open the Index.razor file from the Project page.
- In a BigCommerceConnection object, set the connection string:
BigCommerce authentication is based on the standard OAuth flow. To authenticate, you must initially create an app via the Big Commerce developer platform where you can obtain an OAuthClientId, OAuthClientSecret, and CallbackURL. These three parameters will be set as connection properties to your driver.
Additionally, in order to connect to your BigCommerce Store, you will need your StoreId. To find your Store Id please follow these steps:
- Log in to your BigCommerce account.
- From the Home Page, select Advanced Settings > API Accounts.
- Click Create API Account.
- A text box named API Path will appear on your screen.
- Inside you can see a URL of the following structure: https://api.bigcommerce.com/stores/{Store Id}/v3.
- As demonstrated above, your Store Id will be between the 'stores/' and '/v3' path paramters.
- Once you have retrieved your Store Id you can either click Cancel or proceed in creating an API Account in case you do not have one already.
For example: OAuthClientId=YourClientId; OAuthClientSecret=YourClientSecret; StoreId='YourStoreID'; CallbackURL='http://localhost:33333'
- The code below creates a simple Blazor app for displaying BigCommerce data, using standard SQL to query BigCommerce just like SQL Server.
@page "/" @using System.Data; @using System.Data.CData.BigCommerce; <h1>Hello, world!</h1> Welcome to your Data app. <div class="row"> <div class="col-12"> @using (BigCommerceConnection connection = new BigCommerceConnection( "OAuthClientId=YourClientId; OAuthClientSecret=YourClientSecret; StoreId='YourStoreID'; CallbackURL='http://localhost:33333'")) { var sql = "SELECT FirstName, LastName FROM Customers WHERE FirstName = 'Bob'"; var results = new DataTable(); BigCommerceDataAdapter dataAdapter = new BigCommerceDataAdapter(sql, connection); dataAdapter.Fill(results); <table class="table table-bordered"> <thead class="thead-light"> <tr> @foreach (DataColumn item in results.Rows[0].Table.Columns) { <th scope="col">@item.ColumnName</th> } </tr> </thead> <tbody> @foreach (DataRow row in results.Rows) { <tr> @foreach (var column in row.ItemArray) { <td>@column.ToString()</td> } </tr> } </tbody> </table> } </div> </div>
- Rebuild and run the project. The ADO.NET Provider renders BigCommerce data as an HTML table in the Blazor app.
At this point, you have a BigCommerce-connected Blazor app, capable of working with live BigCommerce data just like you would work with a SQL Server instance. Download a free, 30-day trial and start working with live BigCommerce data in your Blazor apps today.