Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Python Connector Getting Started Guide
With built-in optimized data processing, the CData Python Connector offers unmatched performance for interacting with live data. When issuing complex SQL queries to your application, the driver pushes supported SQL operations, like filters and aggregations, directly to the app and utilizes the embedded SQL engine to process unsupported operations client-side (e.g. often in SQL functions and JOIN operations). In addition, the driver's built-in dynamic metadata querying allows you to work with and analyze application data using native data types.
This article explains how to install the CData Python Connector and create a simple Python script to connect to and query the data.
It is important to note that although this article references the Salesforce Python Connector, the same principles herein can be applied to any of the 250+ data sources that we support.
Download and Install the Connector
First, download the Python Connector from the CData website: https://www.cdata.com/drivers/salesforce/download/python/
Fill in the appropriate contact information.
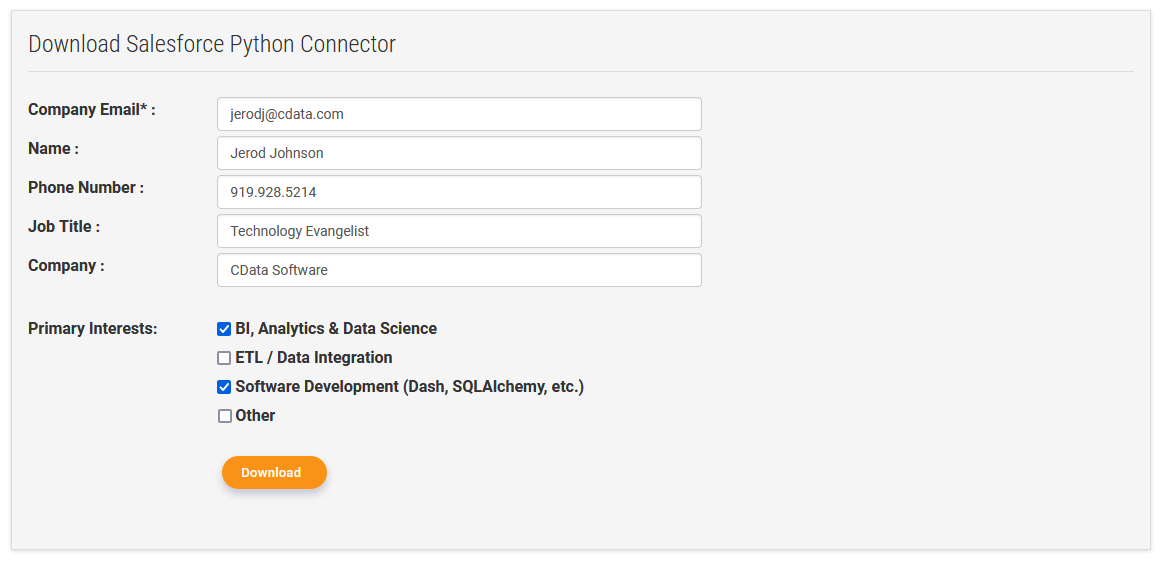
Note: If you are downloading a licensed installer, use your company email address and product key.
Extract the contents of the downloaded ZIP file. Once the contents are extracted, use pip to install the .whl (Windows) or .tar.gz (Linux/Mac) file appropriate for your Python distribution.
Windows Installation
The command below installs the appropriate package for a 64-bit Python 3.10 distribution on a Windows environment (where xxxx is the version number):
pip install PATH\TO\cdata_salesforce_connector-22.0.xxxx-cp310-cp310-win_amd64.whl
Linux/Mac Installation
The command below installs the appropriate package for a Python 3.10 distribution on a Linux environment (where xxxx is the version number):
pip install PATH/TO/cdata_salesforce_connector-22.0.xxxx-python310.tar.gz
Connect to and Query Data
Once the module is installed, you can use it to work with Salesforce data directly from Python. We explain each step in the process below, but include a full script below as well. Start by opening a Python shell by calling python in a terminal.
Import the Module
In the Python shell, import the module:
import cdata.salesforce as salesforce_mod
Note: This import statement is dependent upon the data source to which you are connecting. For example, if you are using the CData Python Connector for Google BigQuery, you would import cdata.bigquery.
Connect to Data
With the module imported, you can use the connect() method to establish a connection to your data source, using a semi-colon (;) separated string of name-value pairs for the required connection properties.
cnxn = salesforce_mod.connect("User=myUser;Password=myPassword;Security Token=myToken;")
To determine the required connection properties, refer to the Establishing a Connection section in Getting Started in the Help documentation.
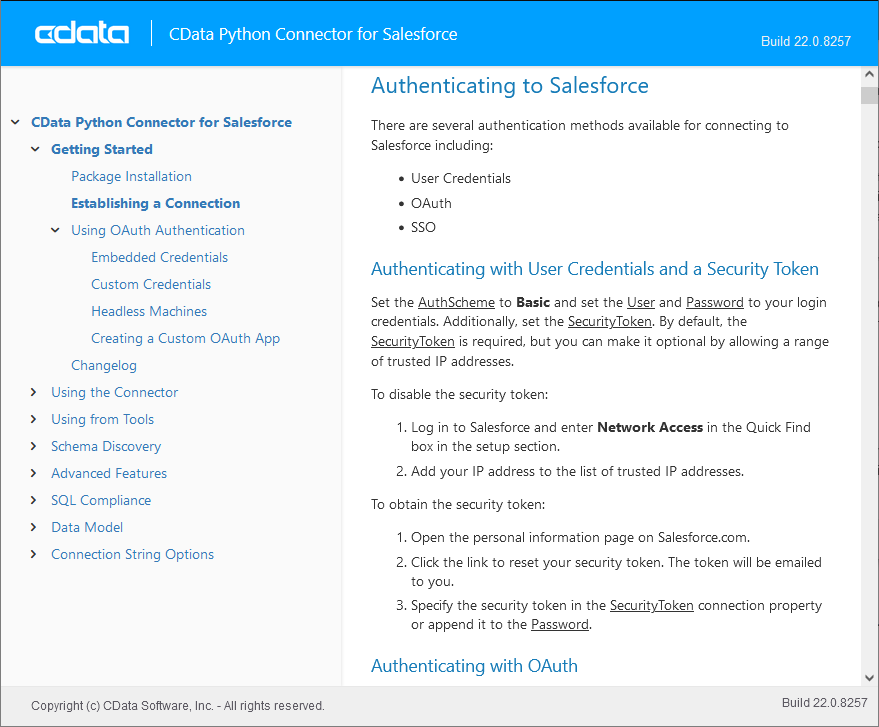
Query Data in Python
After establishing the connection, use the execute function to create a cursor (a Python object which helps execute queries and fetch records from a database).
cursor = cnxn.execute("SELECT * FROM Account")
Use the fetchall function to retrieve the data and store it in a result set.
result_set = cursor.fetchall()
Display the Results
Lastly, iterate over the result set and perform actions and operations on the data. In this case, we simply print each row.
for row in result_set:
print(row)
Full Python Script
The Python script below can be executed in a Python shell, saved in a Python script (a .py file), or run in an IDE.
import cdata.salesforce as salesforce_mod
cnxn = salesforce_mod.connect("User=myUser;Password=myPassword;Security Token=myToken;")
cursor = cnxn.execute("SELECT * FROM Account")
result_set = cursor.fetchall()
for row in result_set:
print(row)
At this point, you have installed a CData Python Connector and used Python to connect to, query, and iterate over Salesforce data. Now you can access live data from 250+ sources in Python scripts and applications, working with your SaaS, Big Data, and NoSQL sources in Python just like you would with a SQL database.
Python Connectors for 250+ Data Sources
For more information on CData's suite of Python Connectors, visit our Python Connector page.
Download a free 30-day trial of the CData Python Connector and get simplified access to your SaaS, Big Data, and NoSQL sources today.